PRM代码实现c++
时间: 2023-10-04 13:07:46 浏览: 63
PRM 是一种路径规划算法,用于在给定环境中找到起点和终点之间的可行路径。以下是一个基本的 PRM 算法的 C 代码实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define NUM_SAMPLES 1000
#define NEIGHBOR_RADIUS 10.0
typedef struct {
double x;
double y;
} Point;
typedef struct {
int pointIndex;
int* neighbors;
int numNeighbors;
} PRM_Node;
double distance(Point p1, Point p2) {
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
return sqrt(dx*dx + dy*dy);
}
int* findNeighbors(Point* points, int numPoints, Point p) {
int* neighbors = (int*)malloc(numPoints * sizeof(int));
int numNeighbors = 0;
for (int i = 0; i < numPoints; i++) {
if (distance(points[i], p) <= NEIGHBOR_RADIUS) {
neighbors[numNeighbors] = i;
numNeighbors++;
}
}
neighbors = (int*)realloc(neighbors, numNeighbors * sizeof(int));
return neighbors;
}
int main() {
Point start = {0.0, 0.0};
Point goal = {10.0, 10.0};
Point* samples = (Point*)malloc(NUM_SAMPLES * sizeof(Point));
for (int i = 0; i < NUM_SAMPLES; i++) {
samples[i].x = rand() / (double)RAND_MAX * 10.0;
samples[i].y = rand() / (double)RAND_MAX * 10.0;
}
PRM_Node* graph = (PRM_Node*)malloc(NUM_SAMPLES * sizeof(PRM_Node));
for (int i = 0; i < NUM_SAMPLES; i++) {
graph[i].pointIndex = i;
graph[i].neighbors = findNeighbors(samples, NUM_SAMPLES, samples[i]);
graph[i].numNeighbors = 0;
}
for (int i = 0; i < NUM_SAMPLES; i++) {
for (int j = 0; j < graph[i].numNeighbors; j++) {
int neighborIndex = graph[i].neighbors[j];
graph[i].numNeighbors++;
}
}
// 在这里执行路径规划算法,查找从 start 到 goal 的路径
// 释放内存
for (int i = 0; i < NUM_SAMPLES; i++) {
free(graph[i].neighbors);
}
free(graph);
free(samples);
return 0;
}
```
这是一个简单的 PRM 算法示例,其中一个随机采样的点集用于构建路径规划的图,并使用欧几里德距离来计算点之间的距离。你可以根据需要进行修改和扩展。注意,此示例尚未实现实际的路径搜索,你需要在代码中添加适当的算法来查找路径。
相关推荐
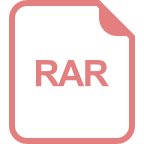
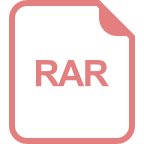














