C++ OPENCV 寻找轮廓,计算轮廓面积,最小外接矩形面积 示例
时间: 2024-09-12 12:12:04 浏览: 81
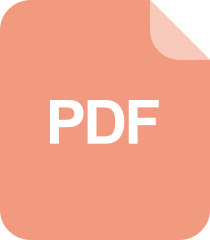
Python opencv 找包含多个区域的最小外接矩形

在C++中,使用OpenCV库进行图像处理时,寻找轮廓并计算其属性非常常见。以下是一个基本示例,展示如何读取图像、找到轮廓、计算轮廓面积以及求最小外接矩形:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/imgproc.hpp>
int main() {
// 1. 读取图片
cv::Mat img = cv::imread("path_to_your_image.jpg", cv::IMREAD_GRAYSCALE); // 灰度图像处理
if (img.empty()) {
std::cout << "Error: Could not read the image." << std::endl;
return -1;
}
// 2. 转换到二值图像便于轮廓检测
cv::threshold(img, img, 127, 255, cv::THRESH_BINARY_INV); // 可调整阈值
// 3. 寻找轮廓
std::vector<std::vector<cv::Point>> contours;
cv::findContours(img, contours, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
// 4. 遍历轮廓,计算面积并找出最小外接矩形
for (const auto& contour : contours) {
int area = cv::contourArea(contour);
cv::Rect boundingRect = cv::boundingRect(contour);
std::cout << "Contour Area: " << area << std::endl;
std::cout << "Bounding Rectangle Area: " << boundingRect.area() << std::endl;
std::cout << "Bounding Rectangle: (" << boundingRect.x << ", " << boundingRect.y << ") - ("
<< boundingRect.width << ", " << boundingRect.height << ")" << std::endl;
}
return 0;
}
```
在这个例子中,`cv::findContours`函数用于查找图像中的轮廓,`cv::contourArea`用于计算轮廓的面积,`cv::boundingRect`则返回轮廓的最小外接矩形。
阅读全文
相关推荐
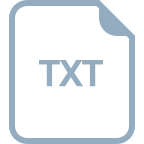
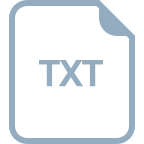




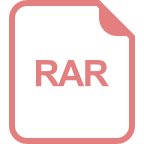
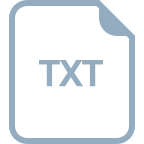
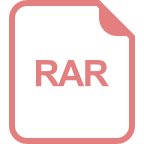
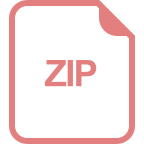
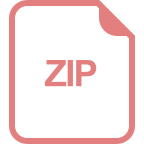
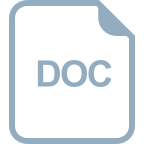
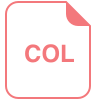
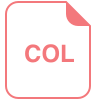
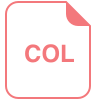


