Functions+can+be+called+from+statements+in+the+body+of+a+loop+and+loops+can+be+called+from+within+th
时间: 2023-12-29 16:25:51 浏览: 21
在Python中,函数可以从循环体内的语句中调用,并且循环可以从函数内部调用。下面是一个示例:
```python
def add_ten(num):
result = num + 10
print("The result is:", result)
# 在循环中调用函数
for i in range(5):
add_ten(i)
# 从函数内部调用循环
def repeat_addition():
for i in range(5):
add_ten(i)
repeat_addition()
```
在上面的示例中,我们定义了一个名为`add_ten`的函数,它将给定的数字加上10并打印结果。然后,我们在循环中调用了这个函数,并且还在另一个函数`repeat_addition`内部调用了循环。
相关问题
datagrip++存储过程
存储过程是一种预编译的数据库对象,它包含了一组SQL语句和控制结构,可以被多次调用。在DataGrip++中,可以通过以下步骤创建和执行存储过程:
1. 创建存储过程
在DataGrip++中,可以使用以下语法创建存储过程:
```sql
CREATE PROCEDURE procedure_name
AS
BEGIN
-- SQL statements
END
```
其中,`procedure_name`是存储过程的名称,`SQL statements`是存储过程中要执行的SQL语句。
2. 执行存储过程
在DataGrip++中,可以使用以下语法执行存储过程:
```sql
EXEC procedure_name
```
其中,`procedure_name`是要执行的存储过程的名称。
3. 查看存储过程
在DataGrip++中,可以使用以下语法查看存储过程的定义:
```sql
SHOW CREATE PROCEDURE procedure_name
```
其中,`procedure_name`是要查看的存储过程的名称。
mybatis+cypher
MyBatis is a Java-based persistence framework that provides mapping between relational databases and Java objects. It helps simplify database access by handling database communication and SQL generation.
On the other hand, Cypher is a query language specifically designed for querying graph databases, such as Neo4j. It allows you to express patterns and relationships in your data in a simple and intuitive way.
If you're looking to use Cypher with MyBatis, it's important to note that MyBatis is primarily designed for relational databases, so it may not have built-in support for Cypher out of the box. However, you can still use MyBatis with Cypher by writing custom SQL queries that execute Cypher statements against a graph database.
To do this, you can use the MyBatis `@SelectProvider` annotation along with a custom SQL provider class. In the SQL provider class, you can write the logic to generate the necessary Cypher queries and execute them against the graph database.
Here's an example of how you can use MyBatis with Cypher:
First, define your Cypher query in the SQL provider class:
```
public class CypherSqlProvider {
public String getNodes() {
return "MATCH (n) RETURN n";
}
}
```
Next, use the `@SelectProvider` annotation in your MyBatis mapper interface:
```
@Mapper
public interface NodeMapper {
@SelectProvider(type = CypherSqlProvider.class, method = "getNodes")
List<Node> getNodes();
}
```
In this example, the `getNodes` method in the `CypherSqlProvider` class generates a Cypher query that matches all nodes in the graph database and returns them. The `@SelectProvider` annotation in the `NodeMapper` interface associates the method with the SQL provider class.
Please note that this is just a basic example, and depending on your specific use case, you may need to modify it accordingly.
I hope this answers your question. Let me know if you have any further queries!
相关推荐
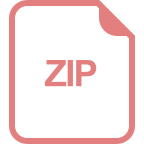
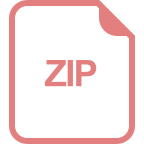
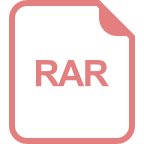












