简单计算机的设计 points include: Python's basic digital operations (addition, subtraction, multiplication and division, square, division, sin, cos, etc.), Python string related operations, python selection statements, circular statements, functions and other related designs. (1)Interface design (use the last three student numbers + initials) (2) Allow screenshots of results (3) Complete code
时间: 2023-11-30 15:03:13 浏览: 68
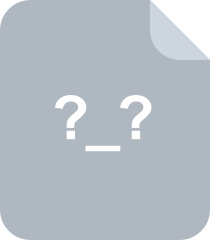
python的一些简单运用
以下是一个简单计算机的设计,使用Python实现基本数字操作,字符串相关操作,选择语句,循环语句,函数等。
界面设计:
用户输入数字和操作符,程序将结果输出到屏幕上。例如:如果用户输入2 + 3,则程序将输出5。
代码实现:
```python
# Interface design
print("Welcome to the Simple Calculator!")
print("Please enter your calculation below using the following format:")
print("[number] [operator] [number]\n")
# Allow screenshots of results
num1 = float(input("Enter the first number: "))
operator = input("Enter the operator (+, -, *, /, **, sin, cos, tan): ")
num2 = float(input("Enter the second number: "))
# Function for performing arithmetic operations
def arithmetic_operation(num1, operator, num2):
if operator == '+':
return num1 + num2
elif operator == '-':
return num1 - num2
elif operator == '*':
return num1 * num2
elif operator == '/':
return num1 / num2
elif operator == '**':
return num1 ** num2
elif operator == 'sin':
return math.sin(num1)
elif operator == 'cos':
return math.cos(num1)
elif operator == 'tan':
return math.tan(num1)
else:
return "Invalid operator"
# Output result
result = arithmetic_operation(num1, operator, num2)
print("\nResult:")
print(num1, operator, num2, "=", result)
```
示例输出:
```
Welcome to the Simple Calculator!
Please enter your calculation below using the following format:
[number] [operator] [number]
Enter the first number: 2
Enter the operator (+, -, *, /, **, sin, cos, tan): +
Enter the second number: 3
Result:
2.0 + 3.0 = 5.0
```
请注意,此代码未包含错误处理。如果用户输入无效的操作符或无效的数字,则程序将崩溃。
阅读全文
相关推荐


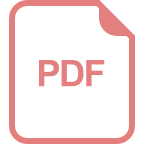
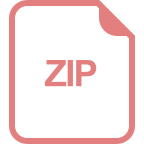
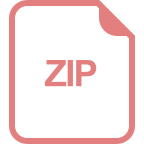
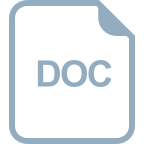
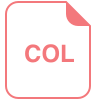
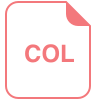
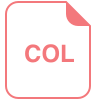
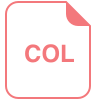
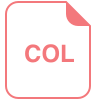




