用c语言输入年、月、日,判断该日子是该年中的第几 天,注意闰年问题。
时间: 2024-09-25 11:01:52 浏览: 20
在C语言中,你可以通过以下几个步骤来编写程序,输入年份、月份和日期,然后判断这一天是否为当年的第几天,同时考虑闰年的因素:
1. 首先,你需要获取用户的输入,并确保它们都在有效范围内(1到31的日期,1到12的月份,非负整数的年份)。
```c
#include <stdio.h>
int main() {
int year, month, day;
printf("请输入年份: ");
scanf("%d", &year);
printf("请输入月份(1-12): ");
scanf("%d", &month);
printf("请输入日期(1-31): ");
scanf("%d", &day);
}
```
2. 接着,计算总天数,考虑到每个月的天数变化以及闰年的规则(闰年2月有29天,其他2月有28天)。
```c
// 定义闰年的判断函数
bool is_leap_year(int year) {
if (year % 4 != 0)
return false;
else if (year % 100 != 0)
return true;
else if (year % 400 == 0)
return true;
else
return false;
}
int days_in_month(int month, int year) {
switch(month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31;
case 4: case 6: case 9: case 11:
return 30;
case 2: // 考虑闰年
if (is_leap_year(year))
return 29;
else
return 28;
default:
return -1; // 输入错误
}
}
int total_days_in_year(int year) {
int sum = 0;
for (int i = 1; i <= 12; ++i) {
sum += days_in_month(i, year);
}
return sum;
}
int main() {
//...
int total_days = total_days_in_year(year);
int actual_day = total_days + day - 1; // 减去前几个月的天数再加上当天
//...
}
```
3. 最后,在main函数中计算实际天数并打印结果。
```c
printf("该日期在该年中的位置: 第%d天\n", actual_day);
return 0;
}
```
相关推荐
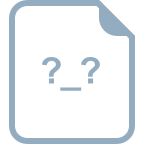
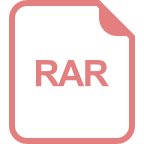














