用C语言定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天,注意闰年问题
时间: 2023-12-26 17:28:02 浏览: 90
以下是用C语言定义一个结构体变量,并计算该日在本年中是第几天的代码:
```c
#include <stdio.h>
typedef struct day {
int year;
int month;
int day;
} DAY;
int calculateDayOfYear(DAY m) {
int i, sum = 0;
int Month[12] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if ((m.year % 4 == 0 && m.year % 100 != 0) || (m.year % 400 == 0)) {
Month[1] = 29;
}
for (i = 0; i < m.month - 1; i++) {
sum += Month[i];
}
return sum + m.day;
}
int main() {
DAY a;
int b;
printf("请输入年月日(以空格分隔):");
scanf("%d %d %d", &a.year, &a.month, &a.day);
b = calculateDayOfYear(a);
printf("今天是%d年的第%d天\n", a.year, b);
return 0;
}
```
相关问题
使用c语言 定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天,注意闰年问题。
下面是一个示例代码,计算该日在本年中是第几天:
```c
#include <stdio.h>
struct Date {
int year;
int month;
int day;
};
int isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
int getDayOfYear(struct Date date) {
int daysInMonth[] = {31, 28 + isLeapYear(date.year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int dayOfYear = date.day;
for (int i = 0; i < date.month - 1; i++) {
dayOfYear += daysInMonth[i];
}
return dayOfYear;
}
int main() {
struct Date date = {2021, 8, 15};
printf("%d/%d/%d is the %dth day of the year.\n", date.year, date.month, date.day, getDayOfYear(date));
return 0;
}
```
这个程序中,我们首先定义了一个 `Date` 结构体,包含年、月、日三个成员变量。然后,我们定义了一个 `isLeapYear` 函数,用于判断一个年份是否是闰年。在 `getDayOfYear` 函数中,我们首先定义了一个 `daysInMonth` 数组,表示每个月的天数,其中二月的天数需要特殊处理。然后,我们通过遍历 `daysInMonth` 数组,计算出给定日期在本年中是第几天。最后,在 `main` 函数中,我们定义了一个 `Date` 类型的变量 `date`,并打印出该日期在本年中是第几天。
定义一个结构体变量(包括年月日)计算该日在本年中是第几天 注意闰年问题 用c语言编写代码
在C语言中,我们可以创建一个结构体来存储日期,并编写一个函数来计算给定日期在当年的位置,考虑闰年的规则。首先,我们需要定义一个结构体`Date`:
```c
#include <stdio.h>
// 结构体定义,包含年、月、日
typedef struct {
int year;
int month;
int day;
} Date;
// 计算闰年的辅助函数
int is_leap_year(int year) {
if (year % 4 != 0)
return 0;
else if (year % 100 != 0)
return 1;
else if (year % 400 != 0)
return 0;
else
return 1;
}
// 按照格里高利历计算某日在本年中的天数
int days_in_month(Date date) {
int days[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 默认非闰年,2月份30天
if (is_leap_year(date.year)) {
days[1] = 29; // 闰年2月份有29天
}
return days[date.month - 1] + date.day;
}
// 主函数来测试
int main() {
Date input_date = {2023, 2, 24};
int total_days = 0;
for (int i = 1; i < input_date.month; ++i) {
total_days += days_in_month({input_date.year, i, 1});
}
total_days += input_date.day; // 加上输入日期本身的天数
printf("在%d年,%d月%d日是今年的第%d天.\n",
input_date.year, input_date.month, input_date.day, total_days);
return 0;
}
```
上述代码中,我们先定义了一个`days_in_month`函数,用于计算给定月份的总天数,然后在主函数中通过累加每个月份的天数来计算总天数。注意,这里假设用户输入的是正确的日期。
阅读全文
相关推荐
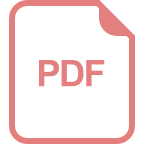
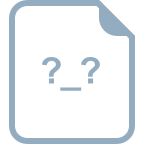













