能否提供一个详细的示例代码,有12片STM32F103每个单片机的串口RS-485通信,包括错误检测、中断管理、超时功能和数据帧解析?同时,请确保这个代码片段包含了必要的库函数调用和初始化步骤。
时间: 2024-10-28 14:12:52 浏览: 55
在STM32F103上实现多个串口RS-485通信,涉及到GPIO配置、USART初始化、中断处理和数据帧解析。这里我将给出一个简化版的示例代码片段,实际项目中可能需要根据具体硬件连接和应用需求做适当调整。假设我们有三个USART用于RS-485通信。
首先,包含必要的头文件:
```c
#include "stm32f10x.h"
#include "stdio.h"
#include "string.h"
#include "usart.h"
#include "rtos.h"
```
然后,初始化相关的USART模块:
```c
void USART_Init(uint32_t USART_ID)
{
GPIO_InitTypeDef GPIO_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_USART2 | RCC_APB2Periph_USART3, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; // RS485_TX for example (configurable)
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource9, GPIO_AF_USART1); // or AF2, AF3 for other USARTs
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; // RS485_RX for example (configurable)
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource10, GPIO_AF_USART1); // or AF2, AF3 for other USARTs
USART_InitStructure.USART_BaudRate = 9600; // Adjust to desired baud rate
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART_ID);
USART_Cmd(USART_ID, ENABLE);
NVIC_InitStructure.NVIC_IRQChannel = USARTx_IRQn; // Replace x with the appropriate USART number (1, 2, or 3)
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
}
```
接下来是简单的数据帧解析和错误检测:
```c
void USART_IRQHandler(uint32_t USART_ID)
{
if(USART_GetITStatus(USART_ID, USART_IT_RXNE) == SET)
{
uint8_t received_char = USART_ReceiveData(USART_ID);
// Check and handle errors here (e.g., CRC check, framing error, etc.)
// For example, assume a simple ASCII frame:
if(received_char != '\n') // End of line is your delimiter
{
// Parse data and potentially store or process it
}
}
}
// Function to send a string over RS485
void SendStringThroughUSART(uint32_t USART_ID, const char *str)
{
while(*str)
{
USART_SendData(USART_ID, *str++);
}
USART_SendData(USART_ID, '\r');
}
```
关于超时功能,可以使用定时器配合中断检查接收状态,这取决于具体的RTOS环境。例如,在FreeRTOS中,你可以创建一个任务定期检查接收缓冲区是否为空。
最后,记得关闭资源:
```c
void USART_DeInit(uint32_t USART_ID)
{
USART_Cmd(USART_ID, DISABLE);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource9, GPIO_AF_OFF); // or AF2, AF3
GPIO_PinAFConfig(GPIOA, GPIO_PinSource10, GPIO_AF_OFF); // or AF2, AF3
GPIO_Init(GPIOA, NULL);
NVIC解散中断;
}
```
阅读全文
相关推荐
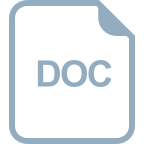
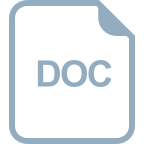
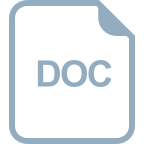
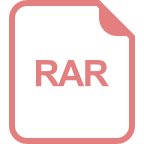
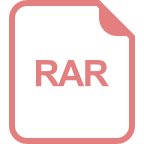
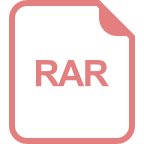
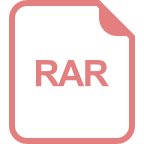
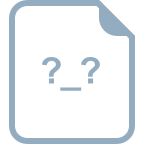
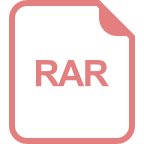
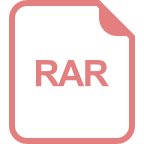
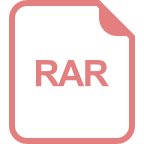
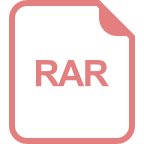
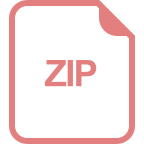
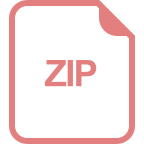
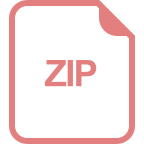
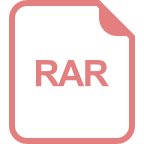
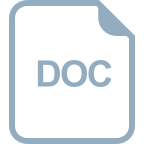
