在pycharm中写段代码用于对文本文件进行情感分析
时间: 2024-12-14 22:19:24 浏览: 13
在PyCharm中进行文本文件的情感分析通常会涉及到自然语言处理(NLP)库,如NLTK、TextBlob或使用深度学习框架如TensorFlow或PyTorch配合transformers。下面是一个简单的例子,我们将使用TextBlob进行基础的情感分析:
```python
from textblob import TextBlob
# 假设你有一个名为'sentiment.txt'的文本文件
def analyze_sentiment(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
# 创建TextBlob对象
blob = TextBlob(content)
# 分析情感极性,范围从-1(负面)到1(正面)
sentiment_polarity = blob.sentiment.polarity
if sentiment_polarity > 0:
print("这是一个积极的文本")
elif sentiment_polarity < 0:
print("这是一个消极的文本")
else:
print("这是一个中立的文本")
analyze_sentiment('sentiment.txt')
```
这个脚本读取文件内容,然后使用TextBlob计算其情感得分,并根据得分判断文本的情感倾向。
相关问题
在pycharm中写段代码用于对文本文件进行情感分析并进行可视化展示
在PyCharm中,我们可以使用Python的一些库如NLTK、TextBlob或Scikit-Learn进行情感分析,然后利用matplotlib或seaborn等库进行结果可视化。这里是一个简单的例子,我们将使用TextBlob库来进行基础的情感分析,并使用matplotlib绘制情感极性分布:
```python
from textblob import TextBlob
import matplotlib.pyplot as plt
# 打开并读取文本文件
with open('your_text_file.txt', 'r') as file:
text = file.read()
# 对每个句子进行情感分析
sentences = text.split('. ')
sentiments = [TextBlob(sentence).sentiment.polarity for sentence in sentences]
# 计算情感分布
positive, neutral, negative = [], [], []
for sentiment in sentiments:
if sentiment > 0:
positive.append(sentiment)
elif sentiment == 0:
neutral.append(sentiment)
else:
negative.append(abs(sentiment))
# 可视化
plt.figure(figsize=(10, 5))
plt.hist([positive, neutral, negative], bins=3, label=['Positive', 'Neutral', 'Negative'], edgecolor='black')
plt.xlabel('Sentiment Polarity')
plt.ylabel('Frequency')
plt.title('Sentiment Analysis Visualization')
plt.legend()
plt.show()
```
请注意将`'your_text_file.txt'`替换为你实际的文件路径。这段代码假设你的文本文件是以句点结尾的句子。如果你想要更复杂的情感分析,可能需要引入预训练的模型,如VADER或BERT。
在pycharm中写段代码用于对csv文件进行情感分析并进行可视化展示
在PyCharm中进行CSV文件的情感分析并可视化,通常需要结合pandas库处理数据,NLTK或TextBlob库进行文本预处理和情感分析,以及matplotlib或seaborn库进行结果的可视化。这里是一个简单的例子:
```python
import pandas as pd
from textblob import TextBlob
import matplotlib.pyplot as plt
# 读取CSV文件
data = pd.read_csv('your_file.csv') # 替换 'your_file.csv' 为你的实际文件路径
# 假设csv有两列,一列为'text',另一列为'label' (例如,0表示负面,1表示正面)
texts = data['text'].tolist()
labels = data['label'].tolist()
# 对文本进行情感分析
sentiments = [TextBlob(text).sentiment.polarity for text in texts]
# 将情感值映射到类别,比如负向、中性和正向
bins = [-1, -0.5, 0, 0.5, 1]
categories = ['Negative', 'Neutral', 'Positive']
sentiments_binned = pd.cut(sentiments, bins=bins, labels=categories)
# 绘制柱状图
plt.figure(figsize=(10, 6))
plt.hist(sentiments_binned, bins=len(categories), edgecolor='black')
plt.xlabel('Sentiment Category')
plt.ylabel('Count')
plt.title('Sentiment Analysis of CSV Data')
plt.xticks(rotation=45) # 为了看清x轴标签
plt.show()
```
阅读全文
相关推荐
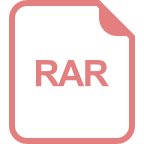
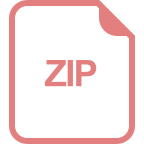
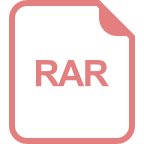

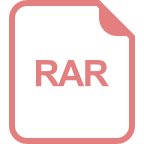
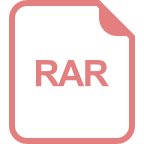
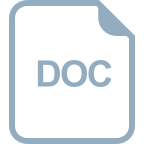
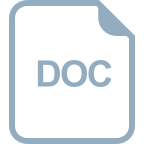
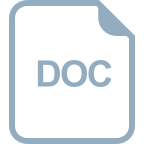
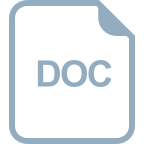
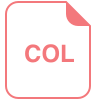
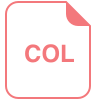
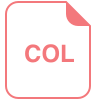
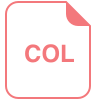
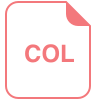
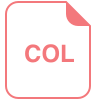
