vue3 ts查看 数据类型
时间: 2024-10-19 15:07:00 浏览: 40
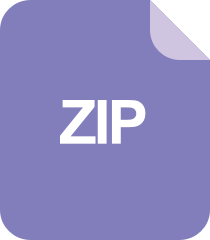
vue3+ts实战项目组件工具库.zip
在 Vue3 中使用 TypeScript 查看数据类型可以通过多种方式实现。以下是一些常用的方法:
1. **使用 TypeScript 的类型声明**:通过定义接口或类型别名来明确变量的类型。例如,如果你有一个用户数据对象,可以定义一个接口来描述它:
```typescript
interface User {
id: number;
name: string;
email: string;
}
const user: User = { id: 1, name: 'John Doe', email: 'john.doe@example.com' };
```
2. **利用 Vue 3 的 `ref` 和 `reactive`**:结合 TypeScript 类型,确保响应式数据的健壮性。例如:
```typescript
import { reactive } from 'vue';
interface DataType {
count: number;
message: string;
}
const data: DataType = reactive({
count: 0,
message: 'Hello World'
});
```
3. **使用 `defineProps` 和 `defineEmits`**:在组合式 API 中,通过 `defineProps` 和 `defineEmits` 来定义组件的 props 和 emits 类型。例如:
```typescript
import { defineProps, defineEmits } from 'vue';
interface PropsType {
title: string;
likes: number;
}
interface EmitsType {
updateLikes(newVal: number): void;
}
const props = defineProps<PropsType>();
const emits = defineEmits<EmitsType>();
```
4. **类型推断**:利用 TypeScript 强大的类型推断功能,减少重复代码。例如,从函数返回值推断变量类型:
```typescript
function getUserData(): User {
return { id: 1, name: 'Jane Doe', email: 'jane.doe@example.com' };
}
const userData = getUserData(); // TypeScript 会自动推断出 userData 的类型为 User
```
5. **JSDoc 注释**:在没有明确的类型声明时,可以使用 JSDoc 注释来说明数据类型。这对于第三方库的使用特别有用:
```typescript
/**
* @param {number} id - The ID of the user.
* @param {string} name - The name of the user.
*/
function createUser(id: number, name: string) {
// Function implementation
}
```
通过这些方法,你可以在 Vue3 项目中有效地查看和使用 TypeScript 数据类型,从而提高代码的可读性和健壮性。
阅读全文
相关推荐
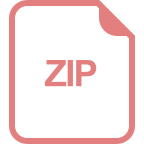
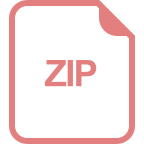

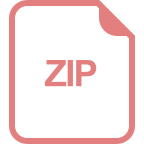













