用java语言写出上述题的答案
时间: 2024-10-17 09:01:49 浏览: 1
由于您提到的问题包含了多个子任务并且每个子任务都需要基于具体的图形数据来进行解答,这里我将提供一种通用的方法来解决这些问题,并给出部分代码示例以帮助您理解如何使用Java实现这些算法。请注意,实际答案还需要依赖于具体的图形输入(即图的具体结构),而以下代码仅为模板性质的内容。
### (1) 最小生成树 - Kruskal与Prim算法
#### 克鲁斯卡尔算法(Kruskal)
- 首先需要定义一个类表示边,并且能够比较它们的权重。
- 使用并查集来避免形成环路。
- 将所有的边按照权重从小到大排序,然后依次加入不形成环的边。
**部分代码示例:**
```java
class Edge implements Comparable<Edge> {
int src, dest, weight;
public int compareTo(Edge compareEdge) {
return this.weight - compareEdge.weight; // Weight comparison
}
}
public class KruskalMST {
static void find(int parent[], int i) { ... } // Find method for Union-Find set
static void union(int parent[], int rank[], int x, int y) { ... } // Union operation
static void KruskalMST(int graph[][]) {
int mstSet[] = new int[V]; // Stores the result (Edges in MST)
Arrays.sort(edges);
int index = 0; // Index of used edges
int e = 0; // An index variable, used for sorted edges
while (e < V - 1) {
Edge next_edge = edgeArray[e++];
int x = find(parent, next_edge.src);
int y = find(parent, next_edge.dest);
if (x != y) {
e++;
mstSet[index++] = next_edge;
union(parent, rank, x, y);
}
}
}
}
```
#### 普里姆算法(Prim)
- 初始化一个数组记录顶点是否被加入到了生成树中。
- 对每一个未访问过的相邻顶点,如果通过当前顶点到达它的距离更近,则更新该顶点的最佳路径。
**部分代码示例:**
```java
public class PrimMST {
private final int V;
PrimMST(Graph G) { ... }
private boolean inMST[]; // Mark vertices included in minimum spanning tree or not
// Function to print constructed MST stored in res[]
void printMST(int parent[], int n, int graph[][])
{
System.out.println("Edge Weight");
for (int i = 1; i < V; i++)
System.out.println(parent[i] + " - " + i + "\t" + graph[i][parent[i]]);
}
// A utility function to find the vertex with minimum distance value, from the set of vertices not yet included in shortest path tree
int minKey(int key[], Boolean mstSet[])
{
// Initialize min value
int min = Integer.MAX_VALUE, min_index = -1;
for (int v = 0; v < V; v++)
if (mstSet[v] == false && key[v] < min)
min = key[v], min_index = v;
return min_index;
}
}
```
### (2) 最短路径 - Dijkstra算法
Dijkstra算法用于求解单源最短路径问题,可以采用优先队列优化实现。
```java
import java.util.PriorityQueue;
public class DijkstraSP {
private final DirectedEdge[] edgeTo; // Shortest paths tree
private final double[] distTo; // Distance of shortest path
private final IndexMinPQ<Double> pq; // Vertices to be relaxed
public DijkstraSP(EdgeWeightedDigraph G, int s) {
...
while (!pq.isEmpty()) relax(G, pq.delMin());
}
private void relax(DirectedEdge e) {
int v = e.from(), w = e.to();
if (distTo[w] > distTo[v] + e.weight()) {
distTo[w] = distTo[v] + e.weight();
edgeTo[w] = e;
if (pq.contains(w)) pq.decreaseKey(w, distTo[w]);
else pq.insert(w, distTo[w]);
}
}
}
```
以上提供的只是基础框架,具体实现时还需考虑细节处理以及异常情况。另外两个关于拓扑排序(topological sort)和关键路径(critical path)的问题,同样可以通过查找相关资料学习其基本原理后编写相应算法实现。希望这能帮到您!
阅读全文
相关推荐
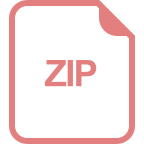
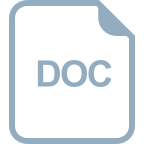
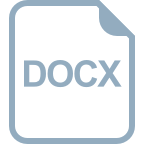















