对71,53,46,84,213,20,16,32,52,108,66,97实现快速排序,并给出c++代码
时间: 2024-09-16 21:06:21 浏览: 36
快速排序是一种常用的排序算法,它基于分治策略,通过一趟排序将待排记录分隔成独立的两部分,其中一部分记录的关键字均比另一部分的关键字小,然后分别对这两部分记录继续进行排序。以下是使用C++实现快速排序的例子,以数组`int arr[] = {71, 53, 46, 84, 213, 20, 16, 32, 52, 108, 66, 97}`为例:
```cpp
#include <iostream>
using namespace std;
// 指定基准值,这里选择第一个元素作为基准
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
// 交换分区后的两个部分,使得小于基准的元素都在基准前面,大于基准的元素在基准后面
int partition(int arr[], int low, int high) {
int pivot = arr[low]; // 基准值
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot; // 将基准放回其最终位置
return low;
}
// 快速排序函数
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1); // 对左侧部分递归排序
quickSort(arr, pi + 1, high); // 对右侧部分递归排序
}
}
int main() {
int arr[] = {71, 53, 46, 84, 213, 20, 16, 32, 52, 108, 66, 97};
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Before sorting: ";
for (int i : arr)
cout << i << " ";
quickSort(arr, 0, n - 1);
cout << "\nAfter sorting: ";
for (int i : arr)
cout << i << " ";
return 0;
}
```
当你运行这个程序,它会按照升序对给定的数组进行排序。如果你需要看到具体的步骤,可以打印数组在每次分割过程中的状态。
阅读全文
相关推荐
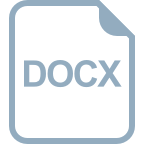
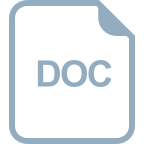
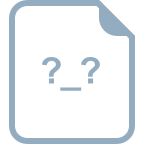

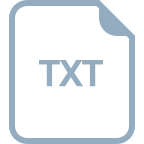
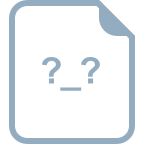
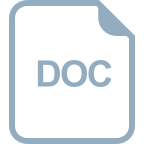
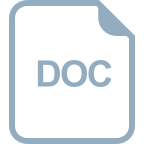
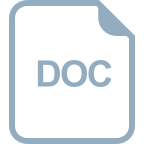
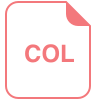








