用java写一个矩形的继承器
时间: 2024-10-16 12:05:56 浏览: 19
在Java中,我们可以创建一个名为Rectangle的类作为Shape的子类,因为形状通常会有几何属性如长度和宽度,而矩形是一种具体的形状。以下是简单的例子:
```java
// 定义一个抽象的Shape类
abstract class Shape {
protected String color; // 共享属性
public Shape(String color) {
this.color = color;
}
// 抽象方法,用于打印形状的基本信息
abstract void display();
}
// 矩形Rectangle类继承自Shape,并实现display()方法
class Rectangle extends Shape {
private double length;
private double width;
public Rectangle(String color, double length, double width) {
super(color);
this.length = length;
this.width = width;
}
// 实现display()方法,显示矩形的信息
@Override
public void display() {
System.out.println("Color: " + color + ", Rectangle with length " + length + " and width " + width);
}
// 可能需要的方法,比如计算面积或周长
public double getArea() {
return length * width;
}
public double getPerimeter() {
return 2 * (length + width);
}
}
// 使用示例
public class Main {
public static void main(String[] args) {
Rectangle rectangle = new Rectangle("blue", 5, 4); // 创建一个蓝色的矩形
rectangle.display(); // 输出矩形信息
System.out.println("Area: " + rectangle.getArea()); // 计算并输出面积
}
}
```
在这个例子中,`Rectangle`类继承了`Shape`类,并覆盖了其中的抽象方法`display()`,同时也添加了自己的特有属性和方法。这体现了多态性,因为你可以用`Shape`类型的引用去操作`Rectangle`实例,而`display()`方法会根据实际类型的不同执行相应的代码。
阅读全文
相关推荐
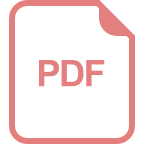
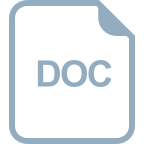
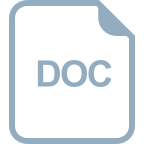
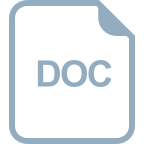














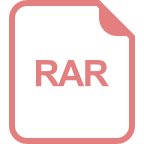