用c语言实现变积分系数pid
时间: 2024-09-18 13:06:42 浏览: 31
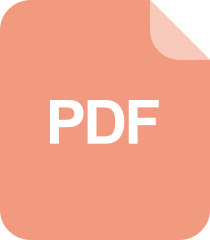
模糊+PID C语言实现
在C语言中实现带变化积分系数的PID控制器(Proportional-Integral-Derivative controller),通常涉及到数学库函数如`math.h`以及自定义的算法。PID控制器的核心部分包含三个组件:
1. **比例(P)**:直接对误差(设定值与当前值之差)进行放大。
2. **积分(I)**:累加过去的误差,用于消除长期趋势。
3. **微分(D)**:对误差的变化率进行控制,减少快速响应过程中的震荡。
如果积分系数随时间变化,你需要维护一个变量来存储这个动态系数,并在每次更新积分项时使用它。以下是一个简化版的PID控制函数示例:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
float Kp; // Proportional gain
float Ki; // Integral gain (with dynamic coefficient)
float KD; // Derivative gain
float prev_error; // Previous error for derivative calculation
float integral; // Integral component
} PID;
// 更新PID控制器
void update_PID(PID* pid, float setpoint, float current_value) {
float error = setpoint - current_value;
pid->integral += pid->Ki * error; // Update integral term with dynamic Ki
// Derivative term
if (pid->prev_error != 0) {
pid->prev_error = error;
float derivative = error / (current_time - previous_time); // Replace 'current_time' and 'previous_time' with actual time measurements
pid->KD *= derivative; // Dynamic derivative gain
}
float output = pid->Kp * error + pid->Ki * pid->integral + pid->KD * error; // Calculate output
// ...do something with 'output'
// Reset integral when it exceeds a limit to avoid windup
if (fabs(pid->integral) > integral_limit) {
pid->integral = signum(error) * integral_limit;
}
}
```
这里假设`current_time`和`previous_time`是当前和上一次计算的时间戳,`integral_limit`是积分饱和的阈值。注意,实际应用中需要处理好动态积分系数的更新策略和时间管理。
阅读全文
相关推荐
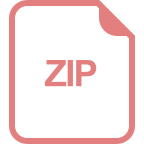
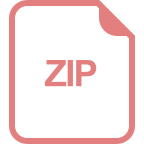
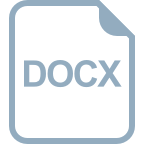
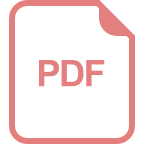
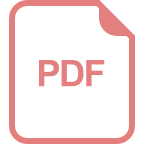











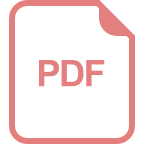
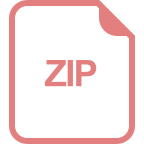