C#读取txt文件某一行并打印
时间: 2024-08-14 10:01:05 浏览: 88
在C#中,你可以使用`StreamReader`类来逐行读取txt文件。以下是一个简单的示例,演示如何读取并打印指定行的内容:
```csharp
using System;
using System.IO;
class Program
{
static void Main()
{
// 文件路径
string filePath = "path_to_your_file.txt";
try
{
// 创建StreamReader实例,并打开文件
using (StreamReader reader = new StreamReader(filePath))
{
// 如果你想读取第n行,可以先获取总行数,然后减去1得到索引
int lineNumber = 5; // 假设你想读第5行
int lineCount = CountLines(reader); // 获取总行数
if (lineNumber <= lineCount)
{
// 读取指定行
string line = reader.ReadLine(lineNumber - 1);
Console.WriteLine("第{0}行内容: {1}", lineNumber, line);
}
else
{
Console.WriteLine("指定的行号超出了文件的实际行数。");
}
}
}
catch (Exception ex)
{
Console.WriteLine($"发生错误: {ex.Message}");
}
}
// 辅助函数,用于计算文件总行数
static int CountLines(StreamReader reader)
{
long currentLine = 0;
string line;
while ((line = reader.ReadLine()) != null)
{
currentLine++;
}
return (int)currentLine;
}
}
```
在这个例子中,你需要将`path_to_your_file.txt`替换为你要读取的txt文件的实际路径。如果你想打印当前行,只需省略行号部分。
阅读全文
相关推荐
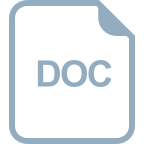
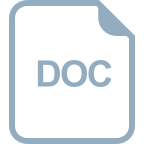
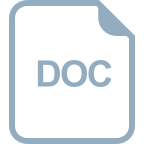
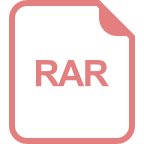
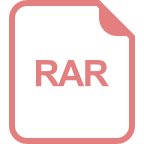
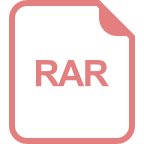
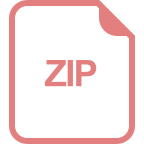
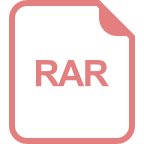
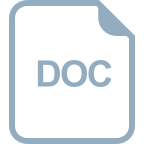
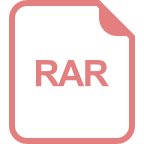
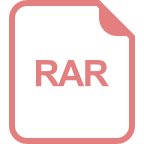







