C语言数组输入一串字符,要求有大小写字母和数字,符号要求显示密码设置成功,否则密码设置失败
时间: 2024-12-23 07:24:32 浏览: 3
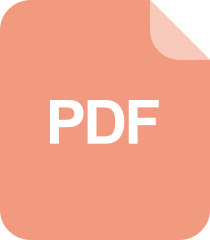
c语言将字符串中的小写字母转换成大写字母
在C语言中,你可以通过循环遍历用户输入的一维字符数组来检查是否包含大小写字母、数字以及指定的符号。首先,你需要定义一个函数,比如`check_password(char[] password)`,来进行这个验证。以下是一个简单的示例:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字符类型的函数
// 定义密码有效性检查函数
int is_valid_char(char c) {
return (isalpha(c) ||.isdigit(c) || c == '*' || c == '@'); // 使用ctype_h库判断字符是否为字母、数字或特定符号
}
// 检查密码并输出结果
void check_password(char password[]) {
int i = 0;
for (; password[i] != '\0'; i++) { // 遍历数组直到遇到空字符'\0'
if (!is_valid_char(password[i])) {
printf("密码设置失败,含有无效字符!\n");
return;
}
}
printf("密码设置成功,包含了大小写字母、数字和指定的符号。\n");
}
int main() {
char user_password[50]; // 假设最大长度为50
printf("请输入密码:");
fgets(user_password, sizeof(user_password), stdin); // 读取用户的输入,包括换行符
// 移除fgets读取的最后一行的换行符
user_password[strcspn(user_password, "\n")] = '\0';
// 检查密码
check_password(user_password);
return 0;
}
```
在这个程序中,我们先定义了一个`is_valid_char`函数用于判断字符是否有效。然后在主函数中,获取用户输入的密码,再调用`check_password`函数进行验证。如果密码满足条件,会显示"密码设置成功";否则,显示"密码设置失败"。
阅读全文
相关推荐
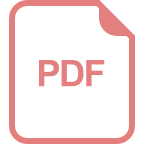
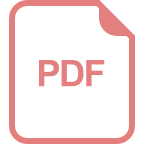
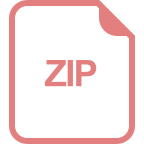
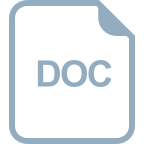
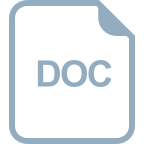
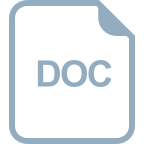
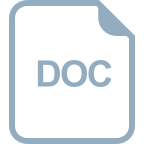
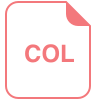
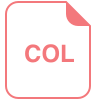
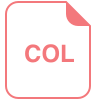







