某比赛有10个评委,每个评委为选手打分(0-10之间),计算成绩的方法:去掉一个最高分和一个最低分,剩余8个评委分数的平均值作为选手的最终得分。请编程输入10个评委的打分,并输出每个评委的编号和所打分数,及选手最后得分。
时间: 2024-12-16 14:32:23 浏览: 17
在Python中,你可以使用列表来存储评委的评分,然后通过排序、截取和求平均的方式得到结果。下面是一个简单的示例程序:
```python
# 初始化评委打分列表
scores = []
# 输入10个评委的打分
for i in range(10):
score = int(input(f"请输入评委{i+1}的打分 (0-10): "))
scores.append(score)
# 对评分进行排序并去掉最高分和最低分
scores.sort()
scores = scores[:-2] + [scores[-1], scores[-2]] # 保持总人数为10
# 计算并输出每个评委的分数
for i, score in enumerate(scores):
print(f"评委{i+1}的打分: {score}")
# 计算并输出选手的最终得分
final_score = sum(scores) / len(scores)
print(f"选手的最终得分为: {final_score}")
```
运行此程序,会依次询问每位评委的打分,并在最后输出每个评委的分数以及选手的最终得分。
相关问题
4.设计一个程序为参加歌手大赛的选手计算最终得分。评委给出的分数是0-10分。选手最后得分:去掉一个最高分,取消一个最低分,计算其余评委打分的平均值。(需确认评委人数并输入每位评委打分)
为了设计这样一个程序,我们需要先明确几个步骤:
1. **获取输入**:
- 首先,询问用户有多少位评委参加了比赛(评委人数)。
- 然后,对每位评委,循环获取他们的评分,直到所有评委的分数都被收集。
2. **处理数据**:
- 创建一个列表来存储所有的评委分数。
- 当收到一个分数时,检查它是否在0到10的范围内,如果不是则提示用户输入有效分数。
3. **去除最高分和最低分**:
- 使用排序算法(如选择排序或快速排序)对分数列表进行排序。
- 删除最大值(最高分)和最小值(最低分)。
4. **计算平均分**:
- 如果剩余评委大于等于2,计算剩余分数的总和除以评委数得到平均分。
- 如果只剩下一个评委,则直接返回他的分数,因为不存在最高分和最低分的概念。
5. **输出结果**:
- 将计算出的平均分作为选手的最终得分显示给用户。
以下是伪代码示例:
```python
def calculate_final_score():
judges = int(input("请输入评委人数: "))
scores = []
for _ in range(judges):
score = float(input(f"请输入第{i+1}位评委的分数(0-10): "))
if score < 0 or score > 10:
print("无效分数,请输入0-10之间的整数。")
continue
scores.append(score)
# 排序并删除最高分和最低分
sorted_scores = sorted(scores)
if len(sorted_scores) >= 2:
final_score = sum(sorted_scores[1:-1]) / (judges - 2)
else:
final_score = sorted_scores[0]
return final_score
final_score = calculate_final_score()
print(f"选手的最终得分为: {final_score}")
```
Python 编程,体育比赛中评委给参赛选手打分,选手得分规则为去掉一个最高分和最低分,然后计算平均得分
在Python编程中,如果你需要模拟体育比赛评委给参赛选手打分的过程,通常可以采用列表或元组来存储每位选手的所有评分,然后通过循环结构去除最高分和最低分,再计算剩余分数的平均值。以下是简单的步骤和示例代码:
1. 首先,定义一个包含所有选手评分的列表或元组,例如:
```python
scores = [90, 85, 95, 92, 88, 97]
```
2. 确定最高分和最低分索引,可以用`max()`和`min()`函数配合`index()`方法找到它们:
```python
if len(scores) > 0:
highest_index = scores.index(max(scores))
lowest_index = scores.index(min(scores))
```
3. 排除最高分和最低分:
```python
filtered_scores = sorted([score for i, score in enumerate(scores) if i != highest_index and i != lowest_index])
```
这里我们先复制了原列表,然后使用列表推导式和条件筛选出除了最高分和最低分之外的其他分数。
4. 计算平均得分:
```python
average_score = sum(filtered_scores) / (len(filtered_scores) if len(filtered_scores) > 0 else 1)
```
注意,如果去除了所有分数后列表为空,则避免除以零的错误。
完整的代码示例:
```python
scores = [90, 85, 95, 92, 88, 97]
# ... (处理最高分和最低分)
filtered_scores = sorted([score for i, score in enumerate(scores) if i != highest_index and i != lowest_index])
if filtered_scores:
average_score = sum(filtered_scores) / len(filtered_scores)
else:
average_score = 0
print("平均得分:", average_score)
阅读全文
相关推荐
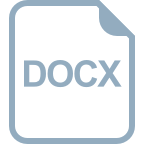
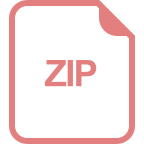
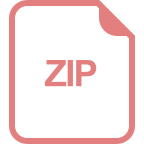













