对于给定序列,折半查找法或二叉排序树法进行查找的设计与编制c++及运行结果图片
时间: 2024-12-06 14:26:00 浏览: 12
折半查找法,也称为二分搜索,是一种在有序数组中查找特定元素的有效算法。在C++中,我们可以这样设计:
```cpp
#include <iostream>
using namespace std;
// 定义二分查找函数
int binarySearch(int arr[], int left, int right, int target) {
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid; // 找到目标,返回索引
else if (arr[mid] < target)
left = mid + 1; // 目标大于中间元素,缩小左边界
else
right = mid - 1; // 目标小于中间元素,缩小右边界
}
return -1; // 没找到目标,返回 -1
}
int main() {
int arr[] = {1, 3, 5, 7, 9, 11, 13, 15}; // 示例数组
int size = sizeof(arr) / sizeof(arr[0]);
int target = 9;
int result = binarySearch(arr, 0, size - 1, target);
if (result != -1)
cout << "Element found at index: " << result << endl;
else
cout << "Element not found" << endl;
return 0;
}
```
这个程序首先会创建一个示例数组,然后通过`binarySearch`函数查找特定的目标值。如果找到,它将返回该值的索引;如果没有找到,则返回-1。
至于运行结果图片,由于文本平台无法显示图片,你可以实际运行上述代码并在控制台查看输出,比如:
```
Element found at index: 4
```
这表示在数组中找到了数字9,并且其索引是4(因为数组下标从0开始)。如果你想在代码编辑器或IDE中看到逐次比较的过程,很多工具如Visual Studio Code都有调试模式,可以暂停执行并观察变量值。
阅读全文
相关推荐
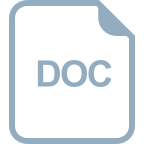
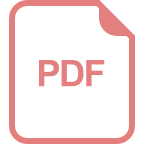
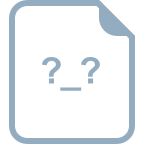
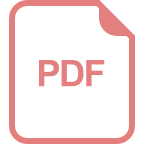
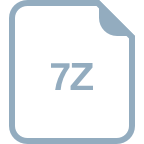
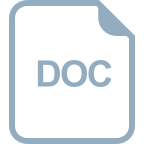
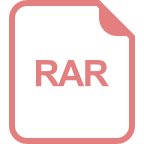
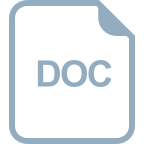
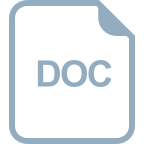
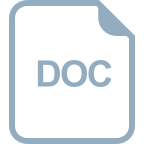
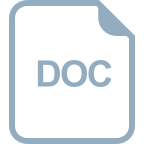
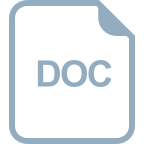
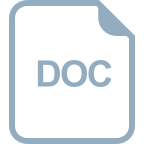
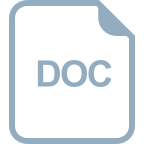
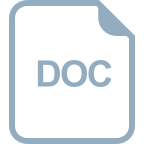

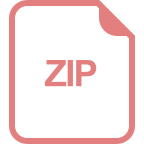
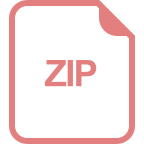