上诉例子中,如何退出waiter线程
时间: 2024-10-23 11:10:14 浏览: 23
在C++中,如果你想让一个线程(例如`waiter`线程)优雅地退出,你可以使用条件变量(`std::condition_variable`)和共享数据(通常是原子标志或互斥量)来同步线程的生命周期。以下是一个简单的示例,展示了如何设置一个退出标志并通知等待的线程:
```cpp
#include <thread>
#include <mutex>
#include <condition_variable>
class Waiter {
public:
std::condition_variable done_cv;
bool is_running; // 停止工作的标志
void start() {
running_thread = std::thread([this] {
while (is_running) {
// 执行线程任务
do_something();
// 检查退出标志
done_cv.wait(std::bind(&Waiter::is_running, this)); // 如果is_running变为false,则唤醒
}
std::cout << "Waiter thread exiting...\n";
});
}
void stop() {
std::unique_lock<std::mutex> lock(mutex);
is_running = false; // 设置为false表示停止
done_cv.notify_all(); // 唤醒所有等待的线程
}
private:
std::thread running_thread;
std::mutex mutex;
};
int main() {
Waiter waiter;
waiter.start();
// ...在其他地方,当需要停止waiter时
waiter.stop();
// 等待主线程结束
if (waiter.running_thread.joinable()) {
waiter.running_thread.join();
}
return 0;
}
```
在这个例子中,`stop()`方法首先锁住`mutex`,然后把`is_running`设为`false`,这会改变条件变量的状态。接着,它调用`done_cv.notify_all()`,这会唤醒所有正在`done_cv.wait()`上的线程。`do_something()`中的循环会在检查到`is_running`为`false`时终止。
在`main`函数中,如果你有控制权,可以调用`waiter.stop()`来结束`waiter`线程,然后使用`running_thread.join()`确保线程真正完成执行并退出。
阅读全文
相关推荐
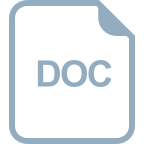
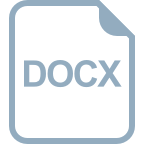
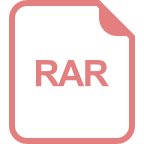















