用c++编写二分递归查找实现在n个有序的数据中查找数据k,并输出k是数据中第几个数
时间: 2024-12-08 07:15:13 浏览: 23
在C++中,你可以使用二分查找算法(也称为折半查找)来在一个已排序的数组`arr`中查找特定元素`k`。二分查找是一种效率较高的搜索算法,适用于数据量较大的情况。以下是二分查找函数的递归版本:
```cpp
#include <iostream>
// 二分查找函数
int binarySearch(int arr[], int low, int high, int k) {
if (high >= low) {
// 计算中间索引
int mid = low + (high - low) / 2;
// 如果中间值就是目标值,直接返回索引
if (arr[mid] == k)
return mid;
// 否则,如果中间值大于目标值,则在左半部分递归查找
else if (arr[mid] > k)
return binarySearch(arr, low, mid - 1, k);
// 否则,在右半部分递归查找
else
return binarySearch(arr, mid + 1, high, k);
}
// 如果没找到目标值,返回-1表示不存在
return -1;
}
int main() {
int n;
std::cout << "Enter the number of elements in the array: ";
std::cin >> n;
int arr[n];
std::cout << "Enter the sorted array: ";
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
int target;
std::cout << "Enter the value to search for: ";
std::cin >> target;
int position = binarySearch(arr, 0, n - 1, target);
if (position != -1)
std::cout << "Element found at index " << position + 1 << ".\n";
else
std::cout << "Element not found.\n";
return 0;
}
```
在这个程序中,用户输入一个排序好的整数数组、需要查找的目标值,然后调用`binarySearch`函数。如果找到了目标值,返回该值在数组中的位置;如果没有找到,返回-1。
阅读全文
相关推荐
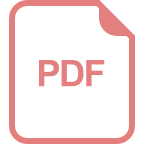
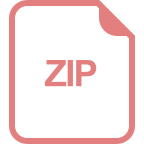
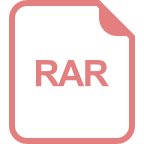
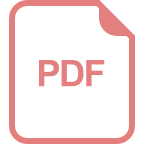
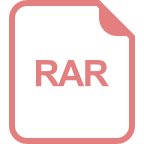
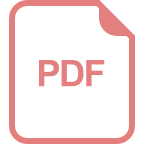
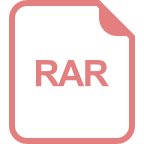
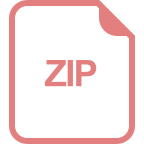
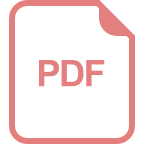
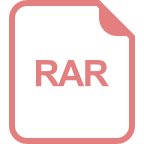
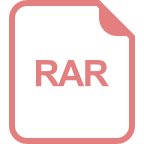
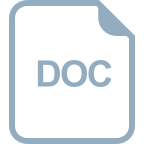
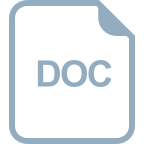
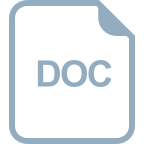
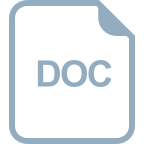
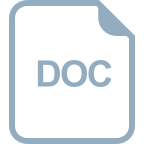
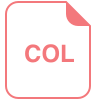
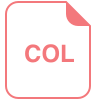
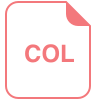