STM32HAL ADC DMA
时间: 2024-02-09 18:59:39 浏览: 93
STM32HAL的ADC DMA是指使用DMA(直接内存访问)的方式来实现STM32微控制器的模数转换(ADC)功能。通过使用DMA,可以实现高效的连续转换和数据传输,减少CPU的负载。
在配置ADC DMA时,需要对DMA进行配置。可以将DMA请求模式设置为循环模式,使得DMA可以不停地进行数据传输。同时,可以设置DMA数据传递方向为外设到内存,以及指定外设地址和内存地址的增加方式,以及每次数据传递的宽度。在配置完成后,只需稍作修改ADC的代码,即可开始使用DMA进行数据转换。
使用DMA进行ADC转换的优势在于可以提高系统效率。当需要多个通道同时进行转换时,使用DMA可以并行进行转换,减少系统资源的占用。尤其是随着通道数量的增加,DMA的优势更加明显。
相关问题
stm32 hal adc dma手动触发
STM32 HAL库提供了一个方便的接口来对ADC进行配置和使用。在使用DMA进行数据传输时,ADC的手动触发模式允许用户在需要时手动触发ADC转换并通过DMA接收转换结果。
要使用ADC的手动触发模式,首先需要配置ADC和DMA的初始化参数。通过调用HAL_ADC_Init()和HAL_ADCEx_Calibration_Start()函数来初始化ADC;通过调用HAL_ADC_Start_DMA()函数来初始化DMA。
在手动触发模式下,我们可以通过调用HAL_ADC_Start()函数来手动触发转换。当该函数被调用时,ADC将开始进行转换,并将转换结果存储在DMA缓冲区中。为了接收转换结果,我们需要在DMA传输完成后使用DMA的中断回调函数。
一般情况下,我们可以通过以下步骤使用手动触发模式:
1. 配置ADC和DMA的初始化参数,包括通道、采样时间、转换分辨率等。
2. 初始化ADC和DMA,使用HAL_ADC_Init()和HAL_ADC_Start_DMA()函数。
3. 在需要的时候,调用HAL_ADC_Start()函数手动触发ADC转换。
4. 等待DMA传输完成的中断发生。
5. 在DMA传输完成的中断回调函数中处理转换结果。
需要注意的是,手动触发模式需要用户根据具体的应用需求来控制转换的时机和频率。通过合适的触发源和触发极性配置,我们可以在需要的时候手动触发ADC转换,并通过DMA接收转换结果。
综上所述,STM32 HAL库的ADC DMA手动触发模式允许用户根据需求手动触发ADC转换,并使用DMA来接收转换结果。通过正确配置和使用相关函数,我们可以灵活地控制ADC的转换和数据传输。
stm32 hal库adc dma配置
STM32 HAL库ADC DMA配置步骤如下:
1. 配置ADC模块:使用HAL_ADC_Init()函数初始化ADC模块,设置采样时间、分辨率、转换模式等参数。
2. 配置DMA模块:使用HAL_DMA_Init()函数初始化DMA模块,设置数据传输方向、数据宽度、传输模式等参数。
3. 配置ADC DMA传输:使用HAL_ADC_Start_DMA()函数启动ADC DMA传输,设置DMA缓冲区地址和大小。
4. 在DMA传输完成中断中处理数据:使用HAL_ADC_ConvCpltCallback()函数,在DMA传输完成中断中处理ADC采样数据。
以上是STM32 HAL库ADC DMA配置的基本步骤,具体实现需要根据具体应用场景进行调整。
阅读全文
相关推荐
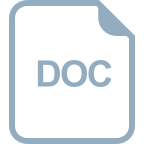
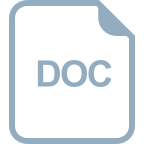
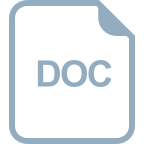
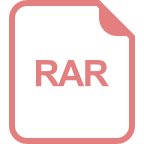
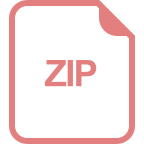




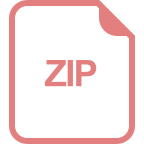
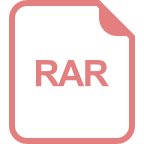
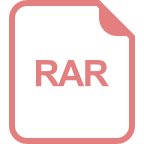




