out = F.avg_pool2d(out, 4)
时间: 2024-04-13 11:25:44 浏览: 20
这行代码使用了`F.avg_pool2d`函数对`out`进行了平均池化操作。`avg_pool2d`函数是PyTorch中的一个函数,用于执行二维平均池化操作。
具体而言,`F.avg_pool2d(out, 4)`表示对输入`out`进行平均池化,池化窗口的大小为4x4。平均池化是一种常用的池化操作,它将输入的每个窗口区域的值取平均,从而减少特征图的尺寸并保留重要的特征。
这行代码可能是用于在深度残差网络中对特征图进行下采样或降维操作。通过对特征图进行平均池化,可以减少特征图的空间尺寸,并且在保留主要特征的同时减少计算量。最终得到的输出将会是尺寸更小的特征图。
相关问题
class ChannelAttention(nn.Module): def __init__(self, in_planes, ratio = 4): super(ChannelAttention, self).__init__() self.avg_pool = nn.AdaptiveAvgPool2d(1) self.max_pool = nn.AdaptiveMaxPool2d(1) self.fc1 = nn.Conv2d(in_planes, in_planes // ratio, 1, bias=False) self.relu1 = nn.ReLU() self.fc2 = nn.Conv2d(in_planes // ratio, in_planes, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): avg_out = self.fc2(self.relu1(self.fc1(self.avg_pool(x)))) max_out = self.fc2(self.relu1(self.fc1(self.max_pool(x)))) out = avg_out + max_out return self.sigmoid(out) 改为tensorflow形式
在 TensorFlow 中,可以使用以下代码来实现相同的通道注意力模块:
```python
import tensorflow as tf
class ChannelAttention(tf.keras.layers.Layer):
def __init__(self, in_planes, ratio=4):
super(ChannelAttention, self).__init__()
self.avg_pool = tf.keras.layers.GlobalAvgPool2D()
self.max_pool = tf.keras.layers.GlobalMaxPool2D()
self.fc1 = tf.keras.layers.Conv2D(in_planes // ratio, kernel_size=1, strides=1, use_bias=False)
self.relu1 = tf.keras.layers.ReLU()
self.fc2 = tf.keras.layers.Conv2D(in_planes, kernel_size=1, strides=1, use_bias=False)
self.sigmoid = tf.keras.layers.Activation('sigmoid')
def call(self, x):
avg_out = self.fc2(self.relu1(self.fc1(self.avg_pool(x))))
max_out = self.fc2(self.relu1(self.fc1(self.max_pool(x))))
out = avg_out + max_out
return self.sigmoid(out)
```
在 TensorFlow 中,我们使用 `tf.keras.layers` 模块来定义网络层,使用 `call()` 方法来实现前向传播。这里的 `GlobalAvgPool2D` 和 `GlobalMaxPool2D` 分别代替了 PyTorch 中的 `AdaptiveAvgPool2d` 和 `AdaptiveMaxPool2d`,它们也可以用来对不同尺寸的输入进行平均池化和最大池化。另外,TensorFlow 中没有 `nn.ReLU` 模块,我们需要使用 `tf.keras.layers.ReLU()` 来替代。其他的代码实现和 PyTorch 版本相同。
用tensorflow的layers.Layer模块改写class ChannelAttention(nn.Module): def init(self, in_planes, ratio = 4): super(ChannelAttention, self).init() self.avg_pool = nn.AdaptiveAvgPool2d(1) self.max_pool = nn.AdaptiveMaxPool2d(1) self.fc1 = nn.Conv2d(in_planes, in_planes // ratio, 1, bias=False) self.relu1 = nn.ReLU() self.fc2 = nn.Conv2d(in_planes // ratio, in_planes, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): avg_out = self.fc2(self.relu1(self.fc1(self.avg_pool(x)))) max_out = self.fc2(self.relu1(self.fc1(self.max_pool(x)))) out = avg_out + max_out return self.sigmoid(out)
以下是使用tensorflow的layers.Layer模块改写的ChannelAttention类:
```
import tensorflow as tf
from tensorflow.keras import layers
class ChannelAttention(layers.Layer):
def __init__(self, in_planes, ratio=4):
super(ChannelAttention, self).__init__()
self.avg_pool = layers.GlobalAveragePooling2D()
self.max_pool = layers.GlobalMaxPooling2D()
self.fc1 = layers.Conv2D(in_planes // ratio, 1, activation='relu', use_bias=False)
self.fc2 = layers.Conv2D(in_planes, 1, use_bias=False)
self.sigmoid = layers.Activation('sigmoid')
def call(self, x):
avg_out = self.fc2(self.fc1(self.avg_pool(x)))
max_out = self.fc2(self.fc1(self.max_pool(x)))
out = layers.add([avg_out, max_out])
return self.sigmoid(out)
```
在这个改写版本中,我使用了tensorflow的layers.Layer模块,并且对应用到了tensorflow中的层。使用layers.GlobalAveragePooling2D()代替了nn.AdaptiveAvgPool2d(1),使用layers.GlobalMaxPooling2D()代替了nn.AdaptiveMaxPool2d(1)。同时,我使用了layers.Conv2D()代替了nn.Conv2d(),并且在第一个卷积层中加入了激活函数。最后,我使用了layers.add()代替了avg_out + max_out。
相关推荐
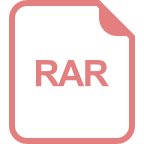









