在数据结构中的顺序栈中如何将中缀转为后缀,用cpp语言写出核心算法
时间: 2024-09-27 08:08:42 浏览: 46
在数据结构中的顺序栈中将中缀表达式转换为后缀(也称逆波兰表示法,RPN),通常会使用到三个栈:一个用于存储操作数,一个用于存储运算符,还有一个临时堆栈用于处理当前遇到的操作符。
核心算法步骤如下:
1. 遍历输入的中缀表达式的每个字符:
- 如果是数字,直接压入操作数栈。
- 如果是左括号,将其压入运算符栈。
- 如果是运算符,检查它的优先级:
- 如果当前是空栈或者栈顶运算符的优先级低于新运算符,直接将当前运算符压入运算符栈。
- 否则,继续弹出栈顶运算符直到找到优先级低于新运算符的运算符,然后将它们依次压回操作数栈。
- 如果是右括号,将栈顶的运算符依次压回操作数栈,直到遇到左括号为止,这时可以移除右括号。
2. 当遍历完所有字符后,如果运算符栈非空,需要将剩余的运算符压入操作数栈。
3. 最后,操作数栈内的序列就是后缀表达式的元素。
以下是一个简单的C++实现示例(假设`isOperator()`函数用于判断字符是否为运算符):
```cpp
#include <stack>
#include <string>
std::string infixToRPN(const std::string &infix) {
std::stack<char> opStack;
std::stack<std::string> numStack;
char current;
for (char& c : infix) {
if (isdigit(c)) { // 数字
numStack.push(c + "");
} else if (c == '(') { // 左括号
opStack.push(c);
} else if (c == ')') { // 右括号
while (!opStack.empty() && opStack.top() != '(') {
numStack.push(opStack.top());
opStack.pop();
}
if (!opStack.empty()) {
opStack.pop(); // 移除左括号
}
} else { // 运算符
while (!opStack.empty() && isOperatorGreater(current, opStack.top())) {
numStack.push(opStack.top());
opStack.pop();
}
opStack.push(current);
}
}
while (!opStack.empty()) {
numStack.push(opStack.top());
opStack.pop();
}
return join(numStack);
}
// 辅助函数,将字符串数组连接成一个字符串
std::string join(std::stack<std::string>& stack) {
std::string result;
while (!stack.empty()) {
result += stack.top();
stack.pop();
}
return result;
}
// 判断运算符优先级,这里仅作为演示,实际应用需替换为合适的比较规则
bool isOperatorGreater(char a, char b) {
// 仅做简单示例,可以根据运算符的实际优先级来实现
return a == '+' || a == '-' || a == '*' || a == '/';
}
```
阅读全文
相关推荐
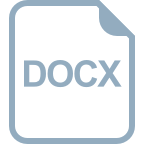
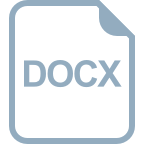

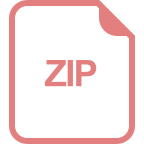
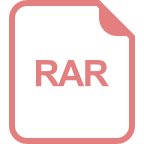
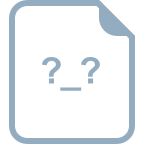
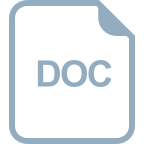
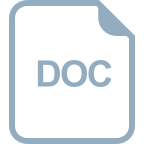
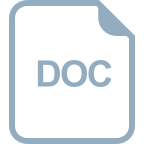
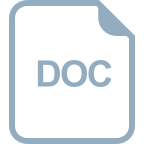




