按照snake文档的要求,根据所提供的snake.py代码框架,实现功能。
时间: 2024-11-03 18:22:11 浏览: 26
根据《Snake.docx》文档提供的指南,以下是实现功能的关键步骤:
### 初始化项目环境
1. **安装pygame**:
```bash
pip3 install pygame
```
### 设置基本参数
1. **屏幕大小和比例**:
- 宽度 (`Width`): 600
- 高度 (`Height`): 600
- 比例 (`Scale`): 30
- 计算公式: `Width = GridWidth * Scale`, `Height = GridHeight * Scale`
2. **颜色定义**:
- 背景颜色 (`BackgroundColor`): (0, 0, 0)
- 文本颜色 (`TextColor`): (255, 255, 255)
- 食物颜色 (`FoodColor`): (255, 0, 0)
- 蛇颜色 (`SnakeColor`): (0, 255, 0)
### 游戏初始化
1. **初始化游戏**:
- 创建 `Game` 类,初始化屏幕和其他必要属性。
- 屏幕属性保存为 `self.Display`。
2. **游戏循环**:
- 监听按键事件,处理退出和方向键。
- 更新游戏状态,绘制帧。
### 实现具体任务
#### Task 1: 单元格上色
1. **实现 `ColourCell` 函数**:
```python
def ColourCell(self, position, color):
x, y = position
rect = pygame.Rect(x * self.Scale, y * self.Scale, self.Scale, self.Scale)
pygame.draw.rect(self.Display, color, rect)
```
2. **在 `DisplayFrame` 中调用 `ColourCell`**:
```python
for cell in snake.Body:
self.ColourCell(cell, self.SnakeColor)
```
#### Task 2: Snake 类
1. **初始化 `Snake` 类**:
```python
class Snake:
def __init__(self):
self.Head = [int(GridWidth / 2), int(GridHeight / 2)]
self.Body = [self.Head]
self.PreviousCell = None
```
2. **在 `Play` 函数中创建蛇实例**:
```python
snake = Snake()
```
#### Task 3: 移动
1. **实现 `Move` 函数**:
```python
def Move(self, direction):
if direction == "Left":
self.Head[0] -= 1
elif direction == "Right":
self.Head[0] += 1
elif direction == "Up":
self.Head[1] -= 1
elif direction == "Down":
self.Head[1] += 1
self.PreviousCell = self.Body[-1].copy()
self.Body.insert(0, self.Head.copy())
self.Body.pop()
```
2. **在 `Play` 中处理按键事件**:
```python
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
snake.Move("Left")
elif keys[pygame.K_RIGHT]:
snake.Move("Right")
elif keys[pygame.K_UP]:
snake.Move("Up")
elif keys[pygame.K_DOWN]:
snake.Move("Down")
```
#### Task 4: 时间和速度
1. **初始化时钟**:
```python
clock = pygame.time.Clock()
GameSpeed = 15
```
2. **修改游戏循环**:
```python
running = True
started = False
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN and not started:
started = True
if started:
snake.Move(current_direction)
# 其他逻辑
self.DisplayFrame()
pygame.display.flip()
clock.tick(GameSpeed)
```
#### Task 5: 食物
1. **实现 `NewFood` 函数**:
```python
def NewFood(self):
self.FoodPosition = [random.randint(0, GridWidth - 1), random.randint(0, GridHeight - 1)]
```
2. **初始化时调用 `NewFood`**:
```python
game.NewFood()
```
3. **在 `DisplayFrame` 中绘制食物**:
```python
self.ColourCell(game.FoodPosition, self.FoodColor)
```
4. **实现 `EatenFood` 函数**:
```python
def EatenFood(self):
return self.Head == game.FoodPosition
```
5. **在游戏循环中检查食物**:
```python
if snake.EatenFood():
game.NewFood()
snake.Body.append(snake.PreviousCell)
score += 1
```
#### Task 6: 游戏结束
1. **实现 `OffScreen` 和 `EatenSelf` 函数**:
```python
def OffScreen(self):
return self.Head[0] < 0 or self.Head[0] >= GridWidth or self.Head[1] < 0 or self.Head[1] >= GridHeight
def EatenSelf(self):
return self.Head in self.Body[1:]
```
2. **在游戏循环中检查游戏结束条件**:
```python
if snake.OffScreen() or snake.EatenSelf():
running = False
```
#### Task 7: 文本显示
1. **在 `DisplayFrame` 中显示分数**:
```python
font = pygame.font.Font(None, 36)
text = font.render(f"Score: {score}", True, self.TextColor)
self.Display.blit(text, (10, 10))
```
2. **在游戏结束时显示信息**:
```python
if not running:
self.Display.fill(self.BackgroundColor)
text = font.render("Game Over", True, self.TextColor)
self.Display.blit(text, (GridWidth // 2 - 50, GridHeight // 2 - 20))
text = font.render(f"Score: {score}", True, self.TextColor)
self.Display.blit(text, (GridWidth // 2 - 50, GridHeight // 2 + 20))
pygame.display.flip()
waiting_for_key = True
while waiting_for_key:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
waiting_for_key = False
running = True
score = 0
snake = Snake()
game.NewFood()
```
通过以上步骤,你可以完成《Snake.docx》文档中描述的所有功能。祝你编程愉快!
阅读全文
相关推荐
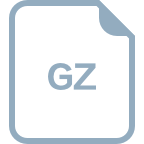










