流ID:对于复杂场景,如Muxing多个输入文件,可能需要提供流索引
时间: 2024-09-11 13:03:09 浏览: 35
在多媒体处理中,特别是当涉及到多个输入文件通过Muxing(合并)操作结合在一起时,流ID(Stream Identifier)就显得十分重要。流ID是一种数字标记,用于标识不同来源(如视频、音频、字幕等)的数据流,在FFmpeg这样的多媒体处理工具中,它们用于区分和管理各个输入流。
当你有多个输入文件,每个文件可能会包含多个单独的数据流(如视频、音频、SRT字幕等)。在Muxing过程中,你需要明确地指定每种数据流应该出现在输出文件中的哪个位置,这就需要用到`-map`选项,并附上流ID。例如,如果你有两个输入文件,文件A有一个视频流和一个音频流,文件B只有音频流,你可以这样做:
```bash
ffmpeg -i input_video_a.mp4 -i input_audio_b.mp3 -c copy -map 0:v:0 -map 1:a:0 output.muxed.mp4
```
在这个例子中,`0:v:0` 表示来自第一个输入文件(video_a)的第一个视频流,`1:a:0` 则表示来自第二个输入文件(audio_b)的第一个音频流。如果没有流ID(例如默认的0),FFmpeg会尝试自动匹配和排列流,但这可能导致意外的结果。
因此,在复杂的Muxing场景中,提供准确的流ID能够保证你的输出文件结构符合预期,避免混淆或丢失数据。
相关问题
Press [q] to stop, [?] for help frame= 900 fps=0.0 q=-1.0 Lsize= 22506kB time=00:00:29.98 bitrate=6147.8kbits/s speed= 331x video:22002kB audio:473kB subtitle:0kB other streams:0kB global headers:0kB muxing overhead: 0.139412%
这是ffmpeg命令在处理视频时的输出信息,其中包含了视频处理的一些参数和统计信息。具体来说,其中包含:
- frame:已处理的帧数
- fps:帧率,即每秒处理的帧数
- q:视频质量,通常为-1.0表示使用默认值
- Lsize:输出文件的大小
- time:已处理的时间
- bitrate:比特率,即输出文件的数据率
- speed:处理速度
- video:视频流的大小
- audio:音频流的大小
- subtitle:字幕流的大小
- other streams:其它流的大小
- global headers:全局头的大小
- muxing overhead:混合开销,即输出文件大小与输入文件大小的比率。
在视频处理过程中,这些信息可以帮助我们了解处理的进度和效果,以便进行调整和优化。在命令行中,按下q键可以停止ffmpeg命令的执行。
qt ffmpeg 推流代码
当使用Qt和FFmpeg进行推流时,你可以使用以下代码示例:
```cpp
#include <QCoreApplication>
#include <QDebug>
#include <QDateTime>
#include <QMutex>
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
#include <libavutil/mathematics.h>
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 初始化FFmpeg库
av_register_all();
avformat_network_init();
AVFormatContext *formatContext = nullptr;
AVOutputFormat *outputFormat = nullptr;
AVStream *stream = nullptr;
AVCodec *codec = nullptr;
AVCodecContext *codecContext = nullptr;
AVPacket packet;
// 配置输入视频参数
QString inputFileName = "input.mp4";
AVFormatContext *inputFormatContext = avformat_alloc_context();
if (avformat_open_input(&inputFormatContext, inputFileName.toUtf8().constData(), nullptr, nullptr) < 0) {
qDebug() << "Could not open input file";
return -1;
}
if (avformat_find_stream_info(inputFormatContext, nullptr) < 0) {
qDebug() << "Could not find stream info";
return -1;
}
// 创建输出上下文
QString outputUrl = "rtmp://your-streaming-server-url";
if (avformat_alloc_output_context2(&formatContext, nullptr, "flv", outputUrl.toUtf8().constData()) < 0) {
qDebug() << "Could not allocate output format context";
return -1;
}
outputFormat = formatContext->oformat;
// 找到视频流索引
for (unsigned int i = 0; i < inputFormatContext->nb_streams; ++i) {
if (inputFormatContext->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
stream = avformat_new_stream(formatContext, nullptr);
if (!stream) {
qDebug() << "Failed allocating video stream";
return -1;
}
codecContext = avcodec_alloc_context3(nullptr);
if (!codecContext) {
qDebug() << "Failed to allocate codec context";
return -1;
}
// 复制输入视频流参数到输出流
avcodec_parameters_to_context(codecContext, inputFormatContext->streams[i]->codecpar);
codecContext->codec_tag = 0;
if (formatContext->oformat->flags & AVFMT_GLOBALHEADER)
codecContext->flags |= AV_CODEC_FLAG_GLOBAL_HEADER;
break;
}
}
// 设置编码器参数
codec = avcodec_find_encoder(AV_CODEC_ID_H264);
if (!codec) {
qDebug() << "Could not find H.264 codec";
return -1;
}
if (avcodec_open2(codecContext, codec, nullptr) < 0) {
qDebug() << "Could not open codec";
return -1;
}
av_dump_format(formatContext, 0, outputUrl.toUtf8().constData(), 1);
// 打开输出URL
if (!(outputFormat->flags & AVFMT_NOFILE)) {
if (avio_open2(&formatContext->pb, outputUrl.toUtf8().constData(), AVIO_FLAG_WRITE, nullptr, nullptr) < 0) {
qDebug() << "Could not open output URL";
return -1;
}
}
// 写文件头部
if (avformat_write_header(formatContext, nullptr) < 0) {
qDebug() << "Could not write output file header";
return -1;
}
// 推流循环
while (true) {
AVStream *inputStream = inputFormatContext->streams[0];
if (av_read_frame(inputFormatContext, &packet) < 0)
break;
packet.stream_index = stream->index;
// 调整时间戳
packet.pts = av_rescale_q_rnd(packet.pts, inputStream->time_base, stream->time_base, (AVRounding)(AV_ROUND_NEAR_INF|AV_ROUND_PASS_MINMAX));
packet.dts = av_rescale_q_rnd(packet.dts, inputStream->time_base, stream->time_base, (AVRounding)(AV_ROUND_NEAR_INF|AV_ROUND_PASS_MINMAX));
packet.duration = av_rescale_q(packet.duration, inputStream->time_base, stream->time_base);
packet.pos = -1;
if (av_interleaved_write_frame(formatContext, &packet) < 0) {
qDebug() << "Error muxing packet";
av_packet_unref(&packet);
break;
}
av_packet_unref(&packet);
}
// 写文件尾部
av_write_trailer(formatContext);
// 清理资源
avcodec_close(codecContext);
avcodec_free_context(&codecContext);
avio_closep(&formatContext->pb);
avformat_free_context(formatContext);
avformat_close_input(&inputFormatContext);
avformat_network_deinit();
return a.exec();
}
```
在代码中,你需要根据实际情况修改输入视频文件名称和输出的 RTMP 流地址。此示例将输入视频文件推流到指定的 RTMP 流地址。请确保FFmpeg库已正确安装并链接到你的Qt项目中。
这只是一个简单的示例,你可以根据需要进行修改和扩展。
阅读全文
相关推荐
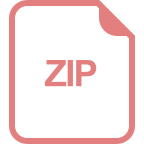
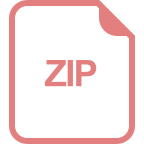
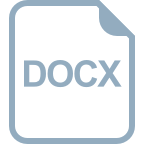
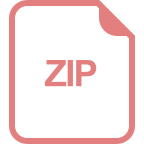
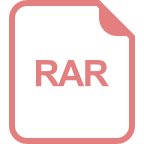
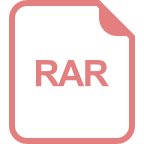
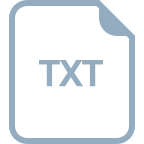
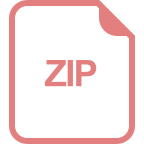
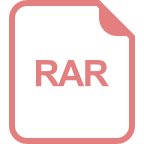
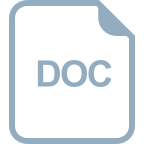
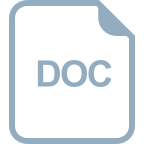
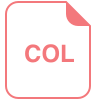



