codeblocks实现音乐暂停
时间: 2023-12-29 07:03:22 浏览: 84
要在CodeBlocks中实现音乐暂停,可以使用winmm库中的PlaySound函数。该函数可以播放WAV文件,并且可以通过设置参数来控制播放方式。要实现暂停,可以使用SND_ASYNC标志来播放声音,并使用SND_PURGE标志来停止声音。具体实现方法如下所示:
```c++
// 在头文件中添加以下代码
#include <Windows.h>
#include <mmsystem.h>
// 在需要播放音乐的地方添加以下代码
PlaySound(TEXT("music.wav"), NULL, SND_FILENAME | SND_ASYNC | SND_LOOP);
// 在需要暂停音乐的地方添加以下代码
PlaySound(NULL, NULL, SND_PURGE);
```
以上代码中,第一个参数是音乐文件的路径,第二个参数是回调函数,第三个参数是播放标志。在播放标志中,SND_FILENAME表示第一个参数是文件名,SND_ASYNC表示异步播放,SND_LOOP表示循环播放。在暂停音乐时,第一个参数设置为NULL,第二个参数同样设置为NULL,第三个参数设置为SND_PURGE。
相关问题
用codeblocks实现爱心代码 用codeblocks实现爱心代码 用codeblocks实现爱心代码高级动态
在Code::Blocks中实现更复杂的动态爱心代码通常是通过图形库,例如Windows API、SFML或Qt等。由于基础版本的Code::Blocks并不直接支持图形绘制,我们这里展示一个简单文字动画的例子,它每秒改变爱心的动态效果,看起来类似爱心在闪烁。我们将使用C++标准库`<chrono>`和`<thread>`来实现定时器和线程。
```cpp
#include <iostream>
#include <thread>
#include <chrono>
#include <random>
// 定义爱心字符数组
const char* heart[] = {"我爱你", "❤️", "💖"};
void display_heart(char* str) {
while (true) {
std::cout << str;
std::this_thread::sleep_for(std::chrono::seconds(1)); // 暂停一秒钟
std::cout.flush();
// 用随机数生成下一个爱心字符串
static std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> dis(0, sizeof(heart) / sizeof(heart[0]) - 1);
str = heart[dis(gen)];
}
}
int main() {
std::thread display(display_heart, heart[0]); // 启动显示线程
// 主线程在此等待,直到按下任意键停止程序
std::cout << "按任意键结束程序...";
getchar();
// 停止显示线程
display.detach(); // 注意:detach不会立即终止线程,而是让它自行结束
return 0;
}
```
这个程序会在新线程里无限循环地显示不同的爱心字符串,然后暂停一秒再换下一种。当你按下任意键,主线程会退出,而爱心线程则会继续运行直到自然结束。
codeblocks实现五子棋
以下是使用 Code::Blocks IDE 实现五子棋的基本步骤:
1. 创建一个新的空项目。
2. 在项目中创建一个新的源文件(例如 main.c)。
3. 在 main.c 文件中包含必要的头文件和宏定义。
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define ROWS 15
#define COLS 15
#define EMPTY 0
#define PLAYER1 1
#define PLAYER2 2
```
4. 创建一个二维数组,表示棋盘。并初始化为 EMPTY。
```c
int board[ROWS][COLS];
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = EMPTY;
}
}
```
5. 创建一个函数,打印当前棋盘。使用“+”表示交叉点,“O”表示玩家1的棋子,“X”表示玩家2的棋子,“.”表示空。
```c
void print_board() {
printf(" ");
for (int i = 0; i < COLS; i++) {
printf("%d ", i+1);
}
printf("\n");
for (int i = 0; i < ROWS; i++) {
printf("%c ", 'a'+i);
for (int j = 0; j < COLS; j++) {
if (board[i][j] == EMPTY) {
printf(". ");
} else if (board[i][j] == PLAYER1) {
printf("O ");
} else if (board[i][j] == PLAYER2) {
printf("X ");
}
}
printf("\n");
}
}
```
6. 创建一个函数,检查某个玩家是否赢得了比赛。检查每一行、每一列和每条对角线是否有五个连续的棋子。
```c
bool check_win(int player) {
// check rows
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS-4; j++) {
if (board[i][j] == player && board[i][j+1] == player && board[i][j+2] == player && board[i][j+3] == player && board[i][j+4] == player) {
return true;
}
}
}
// check columns
for (int i = 0; i < ROWS-4; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == player && board[i+1][j] == player && board[i+2][j] == player && board[i+3][j] == player && board[i+4][j] == player) {
return true;
}
}
}
// check diagonal
for (int i = 0; i < ROWS-4; i++) {
for (int j = 0; j < COLS-4; j++) {
if (board[i][j] == player && board[i+1][j+1] == player && board[i+2][j+2] == player && board[i+3][j+3] == player && board[i+4][j+4] == player) {
return true;
}
}
}
// check anti-diagonal
for (int i = 0; i < ROWS-4; i++) {
for (int j = 4; j < COLS; j++) {
if (board[i][j] == player && board[i+1][j-1] == player && board[i+2][j-2] == player && board[i+3][j-3] == player && board[i+4][j-4] == player) {
return true;
}
}
}
return false;
}
```
7. 创建一个函数,让玩家下棋。玩家输入行和列的坐标,程序检查该位置是否为空,如果是,则将该位置设置为玩家的棋子。如果该位置已经被占用,则提示玩家重新输入。
```c
void play(int player) {
char row, col;
printf("Player %d's turn (row col): ", player);
scanf(" %c %c", &row, &col);
int i = row - 'a';
int j = col - '1';
if (board[i][j] == EMPTY) {
board[i][j] = player;
} else {
printf("This spot is taken! Try again.\n");
play(player);
}
}
```
8. 创建一个主函数,让玩家轮流下棋,直到有一方获胜或棋盘被下满。在每次下棋后,打印当前棋盘,并检查是否有一方获胜。
```c
int main() {
int player = PLAYER1;
while (true) {
print_board();
play(player);
if (check_win(player)) {
printf("Player %d wins!\n", player);
break;
}
player = (player == PLAYER1) ? PLAYER2 : PLAYER1;
bool full = true;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == EMPTY) {
full = false;
break;
}
}
}
if (full) {
printf("Game over! It's a tie.\n");
break;
}
}
return 0;
}
```
这就是实现五子棋的基本步骤。你可以根据需要添加更多的功能和优化。
阅读全文
相关推荐


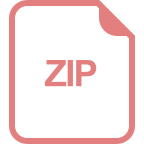
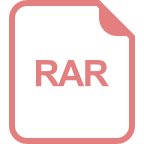
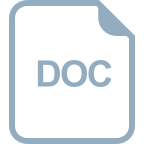
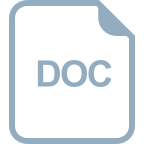






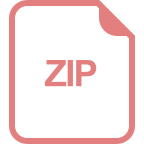