vue3+ts父传子ref
时间: 2023-11-01 09:07:15 浏览: 45
在Vue3中,如果要在父组件中通过ref传递给子组件,需要进行一定的配置。首先,在子组件中声明一个接口,并导出该接口,接口中包含要声明的各个属性和方法。然后,在使用defineExpose时,通过泛型的方式传入该接口(这一步可以省略)。最后,在要使用该组件的文件中导入该声明,并与InstanceType进行联合使用,这样既能获得代码提示,也不会在构建时报错。
具体的实现步骤如下所示:
1. 在子组件中声明一个接口,并导出该接口。接口中包含要声明的属性和方法。
2. 在使用defineExpose时,通过泛型的方式传入该接口。
3. 在要使用该组件的文件中导入该声明,并与InstanceType进行联合使用。使用ref进行绑定,并初始化为null或者null联合上InstanceType<typeof 子组件名> & IClearExposed。
以上是关于在Vue3中通过ref进行父传子的配置方法。
相关问题
vue3+ts 子传父
在Vue 3中,通过使用事件将数据从子组件传递给父组件。以下是在Vue 3和TypeScript中实现子传父的示例:
首先,在子组件中定义一个事件,通过`@emit`修饰符触发该事件,并将需要传递给父组件的数据作为参数进行传递:
```typescript
// ChildComponent.vue
<template>
<button @click="onButtonClick">点击传递数据给父组件</button>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
export default defineComponent({
emits: ['dataToParent'], // 定义一个名为"dataToParent"的事件
setup(_, { emit }) {
const onButtonClick = () => {
const data = 'Hello from child component';
emit('dataToParent', data); // 触发"dataToParent"事件,并传递数据给父组件
};
return {
onButtonClick,
};
},
});
</script>
```
然后,在父组件中监听子组件触发的事件,并处理传递过来的数据:
```typescript
// ParentComponent.vue
<template>
<div>
<p>从子组件接收到的数据:{{ receivedData }}</p>
<child-component @dataToParent="onDataReceived"></child-component>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
export default defineComponent({
data() {
return {
receivedData: '',
};
},
methods: {
onDataReceived(data: string) {
this.receivedData = data; // 处理从子组件传递过来的数据
},
},
});
</script>
```
在父组件中,我们通过`@dataToParent`监听子组件触发的事件,并在`onDataReceived`方法中处理接收到的数据。这样,子组件就可以将数据传递给父组件了。
请注意,上述示例是在Vue 3和TypeScript环境下实现子传父的一种方式。你也可以使用其他方式来实现,例如使用Provide/Inject或Vuex等。
vue3 +ts 父组件调用子组件方法
在 Vue3 + TypeScript 中,可以通过 `ref` 和 `setup()` 函数来实现父子组件之间的方法调用。
首先,在子组件中,需要使用 `defineExpose` 方法将需要暴露给父组件的方法暴露出来:
```typescript
import { defineComponent, defineExpose } from 'vue';
export default defineComponent({
setup() {
const foo = () => {
console.log('foo');
};
const bar = () => {
console.log('bar');
};
// 将 foo 和 bar 方法暴露给父组件
defineExpose({ foo, bar });
return {};
},
});
```
然后,在父组件中,可以使用 `ref` 获取子组件的实例,并调用子组件暴露的方法:
```typescript
import { defineComponent, ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
export default defineComponent({
setup() {
const childRef = ref(null);
const callChildMethod = () => {
childRef.value?.foo();
};
return { childRef, callChildMethod };
},
components: {
ChildComponent,
},
});
```
其中,`childRef` 是一个 `ref` 对象,用来保存子组件的实例。在模板中,需要将子组件的 `ref` 属性绑定到 `childRef` 上:
```html
<template>
<div>
<ChildComponent ref="childRef" />
<button @click="callChildMethod">调用子组件方法</button>
</div>
</template>
```
这样就可以在父组件中调用子组件的方法了。
相关推荐
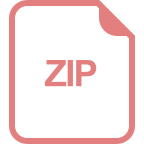
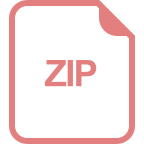
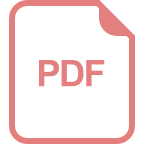












