vue3+ts父组件点击触发子组件弹窗显示隐藏
时间: 2024-01-17 20:19:26 浏览: 195
在Vue3 + TypeScript中,可以通过使用`ref`和`emit`来实现父组件点击触发子组件弹窗的显示和隐藏。
首先,在父组件中,需要定义一个`ref`来控制子组件弹窗的显示和隐藏状态,并在按钮点击事件中触发该状态的改变。同时,需要在子组件上使用`v-if`指令来根据该状态来控制子组件的显示和隐藏。
父组件代码示例:
```vue
<template>
<div>
<button @click="showModal">点击打开弹窗</button>
<child-component v-if="showChildModal" @closeModal="closeModal"></child-component>
</div>
</template>
<script>
import { ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
setup() {
const showChildModal = ref(false);
const showModal = () => {
showChildModal.value = true;
};
const closeModal = () => {
showChildModal.value = false;
};
return {
showChildModal,
showModal,
closeModal
};
}
};
</script>
```
接下来,在子组件中,需要使用`emit`方法来触发一个自定义事件,以便在父组件中关闭弹窗。
子组件代码示例:
```vue
<template>
<div>
<div v-if="visible" class="modal">
<!-- 弹窗内容 -->
<button @click="closeModal">关闭弹窗</button>
</div>
</div>
</template>
<script>
import { ref, defineEmits } from 'vue';
export default {
emits: ['closeModal'],
setup(_, { emit }) {
const visible = ref(false);
const showModal = () => {
visible.value = true;
};
const closeModal = () => {
visible.value = false;
emit('closeModal');
};
return {
visible,
showModal,
closeModal
};
}
};
</script>
```
在上述代码中,父组件通过`@closeModal`监听子组件触发的关闭弹窗事件,并在事件处理函数中关闭弹窗。
阅读全文
相关推荐





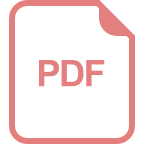
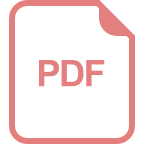
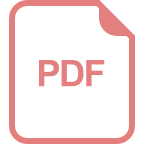
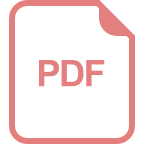
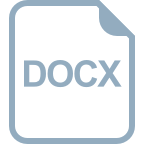
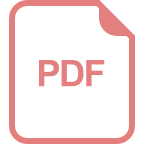
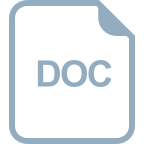
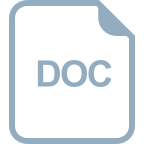


