vue3+ts父组件触发子组件的弹窗组件显示隐藏
时间: 2024-01-17 17:19:14 浏览: 25
在Vue3 + TypeScript中,要实现父组件触发子组件的弹窗组件显示隐藏,可以通过以下步骤来实现:
1. 在父组件中,使用`ref`创建一个响应式变量来控制子组件的显示与隐藏状态。
```typescript
import { ref } from 'vue';
export default {
setup() {
const isModalVisible = ref(false);
const openModal = () => {
isModalVisible.value = true;
};
const closeModal = () => {
isModalVisible.value = false;
};
return {
isModalVisible,
openModal,
closeModal
};
}
};
```
2. 在父组件的模板中,使用`v-model`绑定子组件的显示与隐藏状态。
```html
<template>
<div>
<button @click="openModal">Open Modal</button>
<child-component v-model="isModalVisible"></child-component>
</div>
</template>
```
3. 在子组件中,接收父组件传递的显示与隐藏状态,并根据该状态来控制弹窗的显示与隐藏。
```typescript
import { defineComponent, ref, watch } from 'vue';
export default defineComponent({
props: {
modelValue: {
type: Boolean,
required: true
}
},
emits: ['update:modelValue'],
setup(props, { emit }) {
const isModalVisible = ref(props.modelValue);
watch(
() => props.modelValue,
(newValue) => {
isModalVisible.value = newValue;
}
);
const closeModal = () => {
isModalVisible.value = false;
emit('update:modelValue', false);
};
return {
isModalVisible,
closeModal
};
}
});
```
4. 在子组件的模板中,根据`isModalVisible`变量来控制弹窗的显示与隐藏。
```html
<template>
<div v-if="isModalVisible">
<!-- 弹窗内容 -->
<button @click="closeModal">Close Modal</button>
</div>
</template>
```
相关推荐
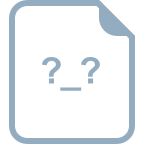
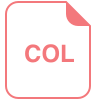
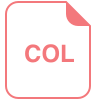












