帮我写一个贪吃蛇小游戏的程序
时间: 2024-10-21 14:04:09 浏览: 43
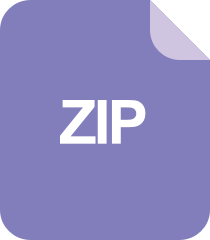
微信小程序-贪吃蛇小游戏
贪吃蛇是一个经典的文本冒险游戏,使用Python来编写一个基本版本的贪吃蛇,我们需要以下步骤:
1. **创建基本环境**:首先,设置一个网格作为蛇的身体部分以及食物的位置。可以使用列表或二维数组表示。
2. **定义角色**:定义蛇的初始位置和移动方向,以及食物的位置。可以使用变量来存储这些信息。
3. **游戏循环**:在一个无限循环里,监听用户的输入来改变蛇的方向。每次循环检查蛇是否撞墙、吃到食物或撞到自己的身体,以此控制游戏结束条件。
4. **更新状态**:基于新的方向,更新蛇的位置和身体长度。如果吃到食物,增加长度,随机生成一个新的食物位置。
5. **绘制游戏界面**:打印出网格,显示蛇的当前位置和食物的位置。可以用简单的字符表示,例如“#”表示墙壁,“O”表示食物,“S”表示蛇头。
6. **异常处理**:处理可能出现的各种错误情况,如边界越界或游戏结束后的退出程序。
下面是一个简化的Python代码示例(注意这只是一个基本框架,完整版会更复杂):
```python
import random
class SnakeGame:
def __init__(self):
self.grid = [['#'] * 10 for _ in range(10)]
self.snake = [(5, 5), (5, 4), (5, 3)] # 初始化蛇
self.food = (random.randint(0, 9), random.randint(0, 9)) # 食物位置
self.direction = 'right'
# 游戏主循环
def game_loop(self):
while True:
self.update_snake()
if self.check_collision() or not self.check_food():
break
print(self.display_grid())
self.listen_input()
# 更新蛇的位置
def update_snake(self):
new_head = self.snake[-1]
if self.direction == 'up':
new_head = (new_head[0], new_head[1] - 1)
elif self.direction == 'down':
new_head = (new_head[0], new_head[1] + 1)
elif self.direction == 'left':
new_head = (new_head[0] - 1, new_head[1])
elif self.direction == 'right':
new_head = (new_head[0] + 1, new_head[1])
self.snake.insert(0, new_head)
# 检查碰撞
def check_collision(self):
return any(new_head in self.snake for head in self.snake[:-1]) or \
new_head in [(0, 0), (9, 9)]
# 检查食物
def check_food(self):
return self.snake[0] == self.food
# 显示网格
def display_grid(self):
grid_str = "\n".join([" ".join(row) for row in self.grid])
return f"Snake: {', '.join(['S'] + ['.']*(len(self.snake)-1))}\nFood: O\n{grid_str}"
# 接收用户输入
def listen_input(self):
direction = input("Enter direction (up/down/left/right): ")
if direction in ['up', 'down', 'left', 'right']:
self.direction = direction
# 创建并运行游戏
game = SnakeGame()
game.game_loop()
```
阅读全文
相关推荐
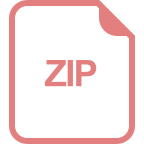
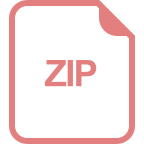














