帮我写一个贪吃蛇小游戏代码
时间: 2024-01-06 15:03:56 浏览: 169
好的,以下是一个简单的贪吃蛇小游戏的 Python 代码,你可以参考一下:
```python
import pygame
import random
# 初始化 Pygame 库
pygame.init()
# 设置游戏窗口的大小
WINDOW_WIDTH = 500
WINDOW_HEIGHT = 500
WINDOW_SIZE = (WINDOW_WIDTH, WINDOW_HEIGHT)
# 设置游戏窗口标题
pygame.display.set_caption("贪吃蛇小游戏")
# 设置游戏背景颜色
BG_COLOR = (255, 255, 255)
# 设置贪吃蛇的大小和速度
SNAKE_SIZE = 10
SNAKE_SPEED = 15
# 定义一个贪吃蛇类
class Snake:
def __init__(self):
self.length = 1
self.positions = [(WINDOW_WIDTH / 2, WINDOW_HEIGHT / 2)]
self.direction = random.choice([pygame.K_UP, pygame.K_DOWN, pygame.K_LEFT, pygame.K_RIGHT])
self.color = (0, 0, 0)
# 获取贪吃蛇头部的位置
def head_position(self):
return self.positions[0]
# 获取贪吃蛇的身体部分的位置
def body_positions(self):
return self.positions[1:]
# 移动贪吃蛇
def move(self):
x, y = self.head_position()
if self.direction == pygame.K_UP:
y -= SNAKE_SIZE
elif self.direction == pygame.K_DOWN:
y += SNAKE_SIZE
elif self.direction == pygame.K_LEFT:
x -= SNAKE_SIZE
elif self.direction == pygame.K_RIGHT:
x += SNAKE_SIZE
self.positions = [(x, y)] + self.positions[:-1]
# 改变贪吃蛇的方向
def change_direction(self, new_direction):
if new_direction == pygame.K_UP and self.direction != pygame.K_DOWN:
self.direction = new_direction
elif new_direction == pygame.K_DOWN and self.direction != pygame.K_UP:
self.direction = new_direction
elif new_direction == pygame.K_LEFT and self.direction != pygame.K_RIGHT:
self.direction = new_direction
elif new_direction == pygame.K_RIGHT and self.direction != pygame.K_LEFT:
self.direction = new_direction
# 增加贪吃蛇的长度
def increase_length(self):
self.length += 1
self.positions.append(self.positions[-1])
# 绘制贪吃蛇
def draw(self, surface):
for position in self.positions:
rect = pygame.Rect(position[0], position[1], SNAKE_SIZE, SNAKE_SIZE)
pygame.draw.rect(surface, self.color, rect)
pygame.draw.rect(surface, BG_COLOR, rect, 1)
# 定义一个食物类
class Food:
def __init__(self):
self.position = (0, 0)
self.color = (255, 0, 0)
self.randomize_position()
# 随机生成食物的位置
def randomize_position(self):
x = random.randint(0, (WINDOW_WIDTH - SNAKE_SIZE) // SNAKE_SIZE) * SNAKE_SIZE
y = random.randint(0, (WINDOW_HEIGHT - SNAKE_SIZE) // SNAKE_SIZE) * SNAKE_SIZE
self.position = (x, y)
# 绘制食物
def draw(self, surface):
rect = pygame.Rect(self.position[0], self.position[1], SNAKE_SIZE, SNAKE_SIZE)
pygame.draw.rect(surface, self.color, rect)
pygame.draw.rect(surface, BG_COLOR, rect, 1)
# 创建游戏窗口
screen = pygame.display.set_mode(WINDOW_SIZE)
# 创建一个贪吃蛇和食物对象
snake = Snake()
food = Food()
# 创建一个 Pygame 的时钟对象,用于控制游戏的帧率
clock = pygame.time.Clock()
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
pygame.quit()
quit()
elif event.key in [pygame.K_UP, pygame.K_DOWN, pygame.K_LEFT, pygame.K_RIGHT]:
snake.change_direction(event.key)
# 移动贪吃蛇
snake.move()
# 判断是否吃到食物
if snake.head_position() == food.position:
snake.increase_length()
food.randomize_position()
# 判断是否撞墙或自撞
if snake.head_position()[0] < 0 or snake.head_position()[0] > WINDOW_WIDTH - SNAKE_SIZE \
or snake.head_position()[1] < 0 or snake.head_position()[1] > WINDOW_HEIGHT - SNAKE_SIZE \
or snake.head_position() in snake.body_positions():
pygame.quit()
quit()
# 绘制游戏界面
screen.fill(BG_COLOR)
snake.draw(screen)
food.draw(screen)
pygame.display.update()
# 控制游戏帧率
clock.tick(SNAKE_SPEED)
```
好了,这个贪吃蛇小游戏的代码已经完成了,你可以直接运行程序来玩一下。如果你有其他问题,可以随时问我哦~
阅读全文
相关推荐
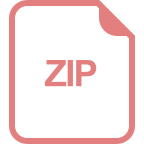
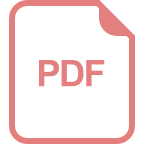
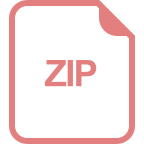









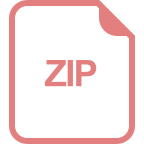
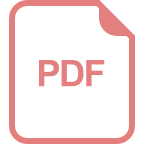
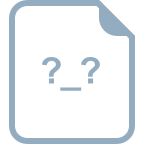
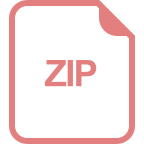
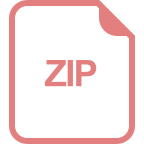
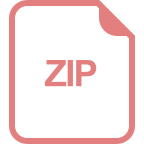