在devc++中,对链表串类型进行定义,用malloc函数为空链表串分配存储空间,建立一个空链表串,用scanf函数输入若干串任意字符串,实现求出该字符串的长度的完整代码
时间: 2024-10-29 15:20:45 浏览: 33
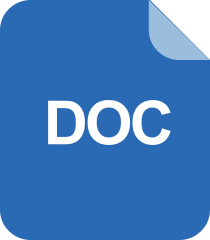
链表 内存的动态存储区
在Dev-C++中,我们可以使用C语言结构体来定义链表,其中包含指向下一个节点的指针以及一个用于存储字符串的数组。首先,我们需要定义链表节点的结构:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct ListNode {
char str[50]; // 假设每个字符串不超过50字符
struct ListNode* next;
} Node;
// 创建一个空链表
Node* createEmptyList() {
Node* head = (Node*) malloc(sizeof(Node));
head->next = NULL;
return head;
}
// 输入字符串并计算长度
void inputAndCalculateLength(Node** head) {
Node* current = *head;
while (current != NULL) {
scanf("%s", current->str); // 输入字符串
int length = strlen(current->str);
printf("String '%s' has a length of %d.\n", current->str, length);
current = current->next;
}
}
int main() {
Node* listHead = createEmptyList();
inputAndCalculateLength(&listHead);
free(listHead); // 清理内存
return 0;
}
```
这个程序首先创建了一个空链表头节点,然后通过`inputAndCalculateLength`函数获取用户输入的字符串,并计算其长度。注意,在结束时别忘了释放动态分配的内存。
阅读全文
相关推荐
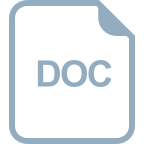
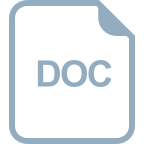



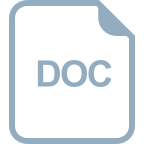








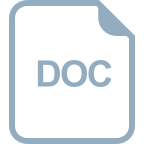