地心惯性坐标系转大地坐标系 java代码
时间: 2024-06-17 20:05:45 浏览: 12
地心惯性坐标系(Earth-Centered Inertial,ECI)是以地球为中心的惯性坐标系。而大地坐标系(Geodetic Coordinates)是以地球表面的形状和大小为基础的坐标系。下面是 Java 代码实现 ECI 坐标系到大地坐标系的转换:
```java
import org.apache.commons.math3.geometry.euclidean.threed.Vector3D;
import org.apache.commons.math3.util.FastMath;
public class EciToGeodetic {
private final double WGS84_A = 6378137.0; // semi-major axis of the Earth
private final double WGS84_B = 6356752.314245; // semi-minor axis of the Earth
private final double WGS84_F = 1.0 / 298.257223563; // Earth flattening coefficient
public GeodeticCoordinate convertEciToGeodetic(Vector3D positionEci, double gmst) {
double x = positionEci.getX();
double y = positionEci.getY();
double z = positionEci.getZ();
double r = FastMath.sqrt(x * x + y * y + z * z);
double f = (WGS84_A - WGS84_B) / WGS84_A;
double e2 = 1.0 - (WGS84_B * WGS84_B) / (WGS84_A * WGS84_A);
double r0 = WGS84_A / FastMath.sqrt(1.0 - e2 * FastMath.sin(gmst) * FastMath.sin(gmst));
double u = FastMath.atan(z / FastMath.sqrt(x * x + y * y));
double l = FastMath.atan(y / x);
double lat;
double lon;
double alt;
do {
lat = u;
double sinLat = FastMath.sin(lat);
double cosLat = FastMath.cos(lat);
double cosLatSq = cosLat * cosLat;
double nu = WGS84_A / FastMath.sqrt(1.0 - e2 * sinLat * sinLat);
double p = r * cosLat - nu * cosLatSq;
double zPrime = r * sinLat + e2 * nu * sinLat;
alt = FastMath.sqrt(p * p + zPrime * zPrime) - nu;
u = FastMath.atan((zPrime / p) * (1.0 - f * nu / (nu + alt)));
} while (FastMath.abs(u - lat) > 1e-12);
lat *= 180.0 / FastMath.PI;
lon = l - gmst;
lon *= 180.0 / FastMath.PI;
if (lon < -180.0) {
lon += 360.0;
} else if (lon > 180.0) {
lon -= 360.0;
}
return new GeodeticCoordinate(lat, lon, alt);
}
public static class GeodeticCoordinate {
private final double latitude;
private final double longitude;
private final double altitude;
public GeodeticCoordinate(double latitude, double longitude, double altitude) {
this.latitude = latitude;
this.longitude = longitude;
this.altitude = altitude;
}
public double getLatitude() {
return latitude;
}
public double getLongitude() {
return longitude;
}
public double getAltitude() {
return altitude;
}
}
}
```
其中,`positionEci` 是在 ECI 坐标系下的位置向量,`gmst` 是格林威治平恒星时。调用 `convertEciToGeodetic` 方法可以将 ECI 坐标系下的位置向量转换为大地坐标系下的经纬度和海拔高度。
相关推荐
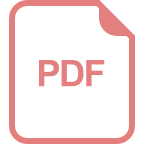
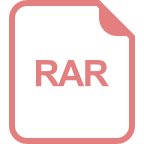














