在地址为"C:\\Users\\11508\\Desktop\\2024-03\\X.bmp"的图像上画两个可移动的圆圈,并实时显示圆心的坐标
时间: 2024-04-15 13:23:44 浏览: 93
要在指定地址的图像上画两个可移动的圆圈,并实时显示圆心的坐标,你可以使用Python的OpenCV库来实现。下面是一个简单的示例代码:
```python
import cv2
# 读取图像
image_path = "C:\\Users\\11508\\Desktop\\2024-03\\X.bmp"
image = cv2.imread(image_path)
# 定义两个圆的初始坐标和半径
circle1_center = (100, 100)
circle1_radius = 20
circle2_center = (200, 200)
circle2_radius = 20
# 创建一个窗口并显示图像
cv2.namedWindow("Image")
cv2.imshow("Image", image)
def mouse_callback(event, x, y, flags, param):
global circle1_center, circle2_center
# 鼠标左键按下时更新圆心坐标
if event == cv2.EVENT_LBUTTONDOWN:
# 更新第一个圆的圆心坐标
if abs(x - circle1_center) <= circle1_radius and abs(y - circle1_center) <= circle1_radius:
circle1_center = (x, y)
# 更新第二个圆的圆心坐标
elif abs(x - circle2_center) <= circle2_radius and abs(y - circle2_center) <= circle2_radius:
circle2_center = (x, y)
# 注册鼠标回调函数
cv2.setMouseCallback("Image", mouse_callback)
while True:
# 在图像上绘制两个圆
image_copy = image.copy()
cv2.circle(image_copy, circle1_center, circle1_radius, (0, 0, 255), -1)
cv2.circle(image_copy, circle2_center, circle2_radius, (0, 255, 0), -1)
# 在图像上显示圆心坐标
cv2.putText(image_copy, f"Circle 1: {circle1_center}", (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
cv2.putText(image_copy, f"Circle 2: {circle2_center}", (10, 60), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
# 显示更新后的图像
cv2.imshow("Image", image_copy)
# 按下ESC键退出循环
if cv2.waitKey(1) == 27:
break
# 关闭窗口
cv2.destroyAllWindows()
```
这段代码会在指定的图像上显示两个圆圈,并且你可以通过鼠标左键拖动圆心来移动圆圈。圆心的坐标会实时显示在图像上。按下ESC键可以退出程序。
阅读全文
相关推荐










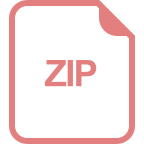
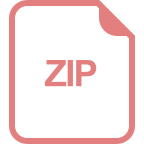
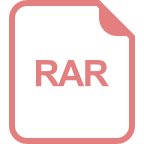
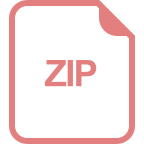