若依框架使用定时任务实现修改数据库的具体代码
时间: 2024-09-30 13:12:15 浏览: 88
若使用Spring Boot框架以及Quartz或其他第三方库如ScheduledExecutorService实现定时任务来更新数据库,这里是一个基本示例,我们将使用Spring的@Scheduled注解配合Repository来操作数据:
首先,假设你有一个`Task`实体类:
```java
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedDate;
@Entity
public class Task {
@Id
private Long id;
private String content;
@CreatedDate
private Date createdAt;
@LastModifiedDate
private Date updatedAt;
// getters and setters
}
```
然后,在一个服务类中添加定时任务方法(例如每小时运行一次):
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
@Service
public class TaskService {
@Autowired
private TaskRepository taskRepository;
// 使用@Scheduled注解标记的定时任务方法
@Scheduled(cron = "0 */1 * * * ?") // 每小时执行一次,cron表达式可以根据需要调整
public void updateDatabase() {
List<Task> tasksToBeUpdated = ...; // 这里可以根据需求查询需要更新的任务
for (Task task : tasksToBeUpdated) {
task.setUpdatedAt(new Date()); // 更新时间戳
taskRepository.save(task); // 通过Repository保存到数据库
}
}
}
```
在这个例子中,你需要配置Spring的`@EnableScheduling`注解启动定时任务,并确保已经启用了Spring Data JPA。
阅读全文
相关推荐
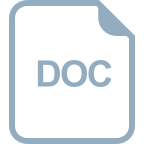
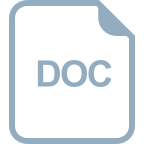
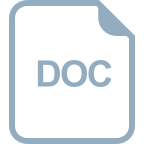
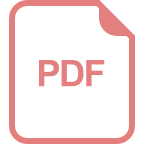
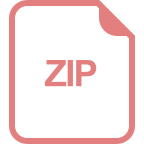
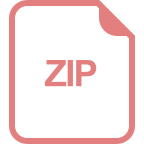
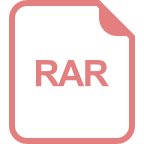
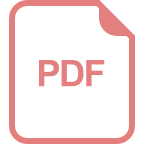
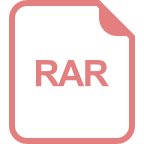
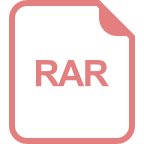
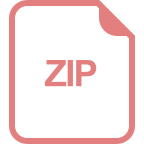
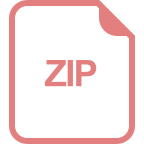
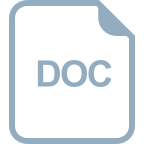
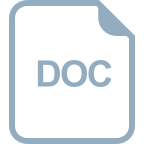
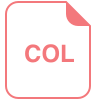



