设二叉树用二叉链表存储,编写程序,将二叉树的所有左右子树交换
时间: 2024-09-01 22:01:36 浏览: 59
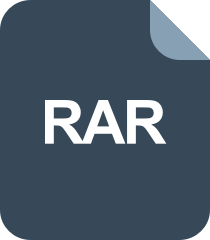
erchashu.rar_交换 二叉树 左右子树_交换左右子树

假设我们有一个二叉链表结构的二叉树,我们可以编写一个递归函数来实现所有节点的左右子树交换。这里是一个简单的Python示例:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def swap_subtrees(root):
if root is None:
return None
# 先交换当前节点的左右子树
temp = root.left
root.left = root.right
root.right = temp
# 然后再递归地交换当前节点的左、右子树的新左右子树
swap_subtrees(root.left)
swap_subtrees(root.right)
# 使用示例
# 假设已经有了一个二叉树root
root = TreeNode(1, TreeNode(2), TreeNode(3))
swap_subtrees(root)
```
这个函数首先检查根节点是否为空,如果是,则返回None。然后交换根节点的左右子树,接着递归地对新的左右子树继续执行此操作。
运行此函数后,整个二叉树的左右子树都会发生交换。
阅读全文
相关推荐
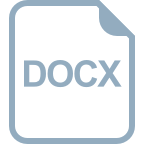
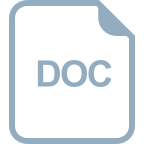















