lstm分类pytorch
时间: 2024-03-22 20:35:44 浏览: 110
LSTM(Long Short-Term Memory)是一种常用的循环神经网络(RNN)变体,它在处理序列数据时能够有效地捕捉长期依赖关系。PyTorch是一个流行的深度学习框架,提供了丰富的工具和函数来构建和训练神经网络模型。
在PyTorch中,使用LSTM进行分类任务的一般步骤如下:
1. 导入必要的库和模块:
```python
import torch
import torch.nn as nn
import torch.optim as optim
```
2. 定义LSTM分类模型:
```python
class LSTMClassifier(nn.Module):
def __init__(self, input_size, hidden_size, num_classes):
super(LSTMClassifier, self).__init__()
self.hidden_size = hidden_size
self.lstm = nn.LSTM(input_size, hidden_size, batch_first=True)
self.fc = nn.Linear(hidden_size, num_classes)
def forward(self, x):
_, (h_n, _) = self.lstm(x)
out = self.fc(h_n[-1])
return out
```
在这个例子中,我们定义了一个LSTM分类器模型,其中包含一个LSTM层和一个全连接层。输入数据的维度为input_size,隐藏状态的维度为hidden_size,输出类别的数量为num_classes。
3. 准备数据:
在训练模型之前,需要准备好训练数据和标签。通常情况下,需要将文本数据转换为数值表示,例如使用词嵌入(Word Embedding)或者词袋模型(Bag of Words)。
4. 定义损失函数和优化器:
```python
model = LSTMClassifier(input_size, hidden_size, num_classes)
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
```
在这个例子中,我们使用交叉熵损失函数和Adam优化器。
5. 训练模型:
```python
for epoch in range(num_epochs):
# 前向传播
outputs = model(inputs)
loss = criterion(outputs, labels)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 打印训练信息
if (epoch+1) % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
```
在每个训练周期中,我们首先进行前向传播计算输出和损失,然后进行反向传播和参数优化。最后,我们可以选择性地打印训练信息。
6. 进行预测:
```python
with torch.no_grad():
outputs = model(inputs)
_, predicted = torch.max(outputs.data, 1)
```
在预测阶段,我们使用训练好的模型对新的输入数据进行预测,并根据输出结果选择最可能的类别。
阅读全文
相关推荐
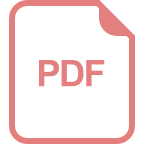
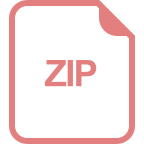















