在python中将sql数据集处理为json数据
时间: 2024-11-30 10:22:24 浏览: 5
在Python中,将SQL查询结果转换为JSON数据通常需要通过连接数据库、执行SQL查询并将其转化为适合的数据结构,最后再使用`json`模块进行序列化。以下是一个简单的步骤:
1. **导入必要的库**:
```python
import sqlite3
import json
```
2. **连接到SQLite数据库**(如果使用的是SQLite):
```python
conn = sqlite3.connect('your_database.db')
cursor = conn.cursor()
```
3. **执行SQL查询**:
```python
query = "SELECT * FROM your_table"
cursor.execute(query)
results = cursor.fetchall()
```
4. **数据转化为列表或其他适合作为字典键值对的形式**:
```python
data_list = [dict(zip(cursor.description, row)) for row in results]
```
这里使用了`zip()`函数将元组拆分成键值对,并用`dict()`创建字典。
5. **将数据转换为JSON**:
```python
json_data = json.dumps(data_list)
```
6. **关闭连接**:
```python
conn.close()
```
完整的示例代码可能会像这样:
```python
import sqlite3
import json
# ... (上述连接数据库的部分)
query = "SELECT * FROM your_table"
cursor.execute(query)
results = cursor.fetchall()
data_list = [dict(zip([col[0] for col in cursor.description], row)) for row in results]
json_data = json.dumps(data_list)
print(json_data)
```
阅读全文
相关推荐
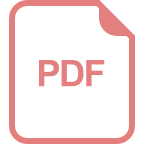
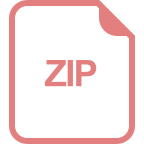
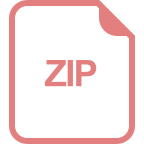
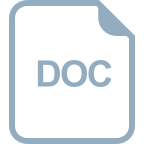
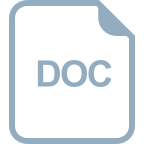
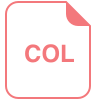
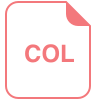
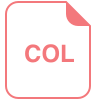
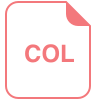
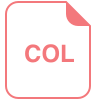
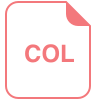
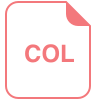
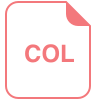
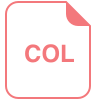
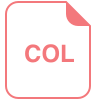
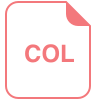
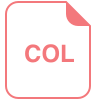
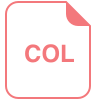
