通过画像素点实现三角形类,C++
时间: 2024-12-27 22:31:53 浏览: 5
在C++中,如果你想要通过像素点来实现一个简单的三角形类,你可以利用计算机图形学的基本原理,特别是位图或像素数组的概念。以下是一个基本的示例,展示如何创建一个简单的二维像素三角形类:
```cpp
#include <vector>
class Triangle {
private:
int x1, y1, x2, y2, x3, y3; // 三角形三个顶点的坐标
std::vector<std::pair<int, int>> pixels; // 存储每个像素点
public:
Triangle(int a, int b, int c, int d, int e, int f) : // 构造函数,传入三角形三个边的两个端点
x1(a), y1(b), x2(c), y2(d), x3(e), y3(f) {
fillPixels(); // 计算并存储像素点
}
void fillPixels() {
if (x1 == x2 && x2 == x3) { // 简单垂直线处理
for (int i = std::min(y1, y3); i <= std::max(y1, y3); ++i) {
pixels.push_back({x1, i});
}
} else if (y1 == y2 && y2 == y3) { // 简单水平线处理
for (int i = std::min(x1, x3); i <= std::max(x1, x3); ++i) {
pixels.push_back({i, y1});
}
} else { // 对于一般三角形,使用Bresenham算法计算像素
int steep = abs(y2 - y1) > abs(x2 - x1);
if (steep) {
std::swap(x1, y1);
std::swap(x2, y2);
std::swap(x3, y3);
}
int dx = x2 - x1;
int dy = abs(y2 - y1);
int error = dx / 2;
int y = y1;
for (int x = x1; x <= x2; ++x) {
pixels.push_back({x, y});
if (steep) {
pixels.push_back({y, x});
}
if (error <= 0) {
y += dy;
error += dx;
} else {
error -= dy;
}
}
}
}
// 其他可能的方法如:绘制、检查是否包含某一点等...
};
阅读全文
相关推荐



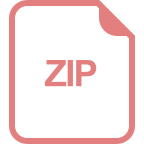















