C++实现多项式校验和crc4,初值为0101
时间: 2024-09-07 13:06:24 浏览: 56
在C++中实现CRC-4(循环冗余检查)算法,特别是对于多项式的校验,通常涉及到位操作和模运算。CRC-4是一种简单的CRC变种,其生成多项式通常是x^4 + x + 1。以下是基本步骤:
1. 初始化CRC寄存器(也称为CRC polynomial register)为初始值0101,即二进制1001。
```cpp
unsigned int initial_crc = 0b1001; // CRC寄存器的初始值
```
2. 对于每个输入字节,你需要将它与当前CRC值异或(XOR),然后对生成多项式做模运算。这里我们假设输入数据为`input_data`数组,遍历每个字节`byte`:
```cpp
for (const auto byte : input_data) {
unsigned int shifted_crc = initial_crc >> 8; // 移位以便接受新字节
shifted_crc ^= byte;
// 应用CRC计算公式(模4)
shifted_crc ^= ((shifted_crc & 0xF0) >> 4); // 第一位
shifted_crc ^= (shifted_crc << 1) & 0x0F; // 下移并与生成多项式相乘
// 更新CRC寄存器
initial_crc = shifted_crc;
}
```
3. 最终的CRC值就是CRC寄存器的内容。
注意,这个示例是一个简化版本,实际应用中可能需要处理特殊情况,例如奇偶校验、结束标志等。下面是完整的函数模板:
```cpp
template <typename T>
unsigned int crc4(const std::array<T, N>& data)
{
unsigned int initial_crc = 0b1001;
for (const auto& byte : data) {
//...
}
return initial_crc;
}
// 使用示例
std::array<unsigned char, 5> input_data = {1, 2, 3, 4, 5};
unsigned int final_crc = crc4(input_data);
```
阅读全文
相关推荐
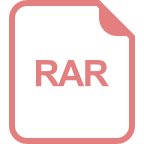
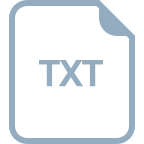
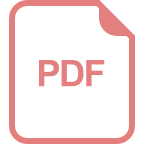
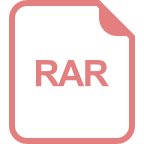
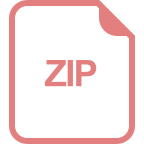
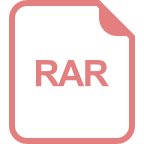
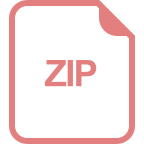
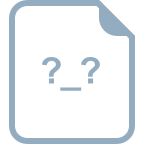
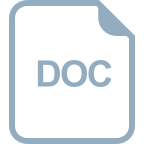
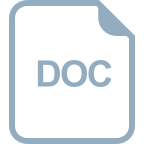
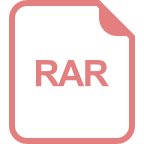
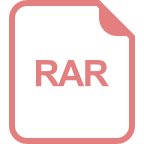
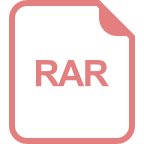
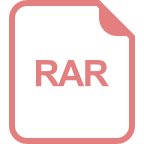