unordered_map自定义哈希函数并传参
时间: 2024-09-29 20:02:51 浏览: 15
`unordered_map` 是 C++ 标准库中的关联容器,它内部使用哈希表实现,提供了快速的查找速度。如果想要在 `unordered_map` 中使用自定义的哈希函数,通常需要提供两个东西:
1. **哈希函数** (Hash Function):这是计算键值哈希码的关键。你需要实现一个接受任意类型键值并返回整数哈希值的函数模板。例如,对于用户自定义类型 T,可以创建一个 `std::hash<T>` 的特化版本。
```cpp
template<>
struct hash<MyCustomType> {
std::size_t operator()(MyCustomType const& value) const {
// 这里编写自定义的哈希计算逻辑,例如基于某些字段的组合
return /*计算哈希值*/;
}
};
```
2. **等价函数** (Equality Comparison Function):如果键可能会冲突,还需要提供一个用于比较键是否相等的函数。通常这个函数会默认是 `<algorithm>` 库中的 `equal_to<>`,但如果需要自定义比较规则,也可以自定义一个模板同名函数。
```cpp
bool equal(MyCustomType const& a, MyCustomType const& b) {
// 这里编写自定义的键值相等判断逻辑
return a.my_field == b.my_field; // 假设 "my_field" 是你要比较的字段
}
template <>
struct equal_to<MyCustomType> {
bool operator()(MyCustomType const& a, MyCustomType const& b) const {
return equal(a, b);
}
};
```
然后在创建 `unordered_map` 时,你可以将这两个函数作为模板参数传递进去:
```cpp
unordered_map<MyCustomType, Value, hash<MyCustomType>, equal_to<MyCustomType>> my_map;
```
相关推荐
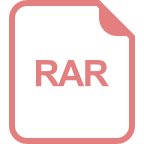
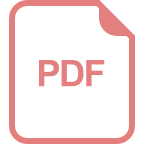
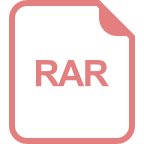














