unordered_map 自定义 hash
时间: 2024-01-12 08:22:18 浏览: 89
unordered_map是C++标准库中的一个关联容器,它使用哈希表来实现键值对的存储和查找。如果我们想要在unordered_map中使用自定义类型作为键,我们需要提供一个自定义的哈希函数来计算键的哈希值。
下面是一个示例,展示了如何在unordered_map中使用自定义哈希函数:
```cpp
#include <iostream>
#include <unordered_map>
#include <string>
struct Person {
std::string name;
int age;
Person(const std::string& n, int a) : name(n), age(a) {}
};
struct PersonHasher {
std::size_t operator()(const Person& p) const {
std::size_t res = 17;
res = res * 31 + std::hash<std::string>()(p.name);
res = res * 31 + std::hash<int>()(p.age);
return res;
}
};
int main() {
std::unordered_map<Person, int, PersonHasher> myMap;
Person p1("Alice", 25);
Person p2("Bob", 30);
myMap[p1] = 100;
myMap[p2] = 200;
std::cout << "Value for p1: " << myMap[p1] << std::endl; // 输出:100
std::cout << "Value for p2: " << myMap[p2] << std::endl; // 输出:200
return 0;
}
```
在上面的示例中,我们定义了一个名为Person的自定义类型,并提供了一个名为PersonHasher的自定义哈希函数。在unordered_map的声明中,我们使用了Person作为键类型,并指定了PersonHasher作为哈希函数。
在PersonHasher中,我们重载了operator()运算符,根据Person对象的name和age成员变量计算哈希值。我们使用std::hash来计算每个成员变量的哈希值,并将它们组合起来得到最终的哈希值。
通过这种方式,我们可以在unordered_map中使用自定义类型作为键,并使用自定义的哈希函数来计算键的哈希值。
阅读全文
相关推荐
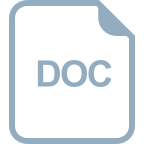
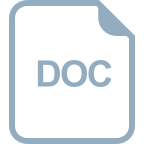
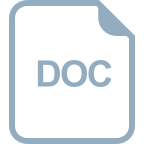


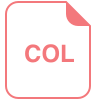
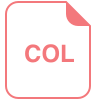







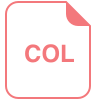
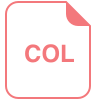
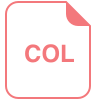

