unordered_map三维索引
时间: 2024-02-05 09:09:23 浏览: 25
unordered_map是C++标准库中的一个关联容器,它提供了一种键值对的映射关系。在unordered_map中,每个键都是唯一的,而值可以重复。通过使用键来访问对应的值,unordered_map可以实现高效的查找操作。
三维索引是指在一个三维空间中,通过三个维度的值来唯一标识一个元素。在使用unordered_map进行三维索引时,可以将三个维度的值组合成一个自定义的结构体或者使用std::tuple作为键,将对应的值作为unordered_map中的值。
下面是一个示例代码,演示了如何使用unordered_map进行三维索引:
```cpp
#include <iostream>
#include <unordered_map>
#include <tuple>
struct Index {
int x;
int y;
int z;
bool operator==(const Index& other) const {
return std::tie(x, y, z) == std::tie(other.x, other.y, other.z);
}
};
namespace std {
template <>
struct hash<Index> {
size_t operator()(const Index& index) const {
return std::hash<int>()(index.x) ^ std::hash<int>()(index.y) ^ std::hash<int>()(index.z);
}
};
}
int main() {
std::unordered_map<Index, int> map;
// 添加元素
Index index1{1, 2, 3};
map[index1] = 10;
// 查找元素
Index index2{1, 2, 3};
if (map.find(index2) != map.end()) {
std::cout << "找到了对应的值:" << map[index2] << std::endl;
} else {
std::cout << "未找到对应的值" << std::endl;
}
return 0;
}
```
在上面的示例代码中,我们定义了一个名为Index的结构体,用来表示三维索引。为了在unordered_map中使用Index作为键,我们需要重载Index的operator==函数,并在std命名空间中定义一个hash模板特化,用来计算Index的哈希值。
通过使用unordered_map和自定义的Index结构体,我们可以实现对三维空间中元素的快速查找和访问。
相关推荐
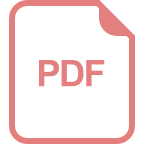
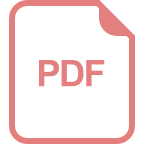














