.net 计算字符串16位CRC校验码
时间: 2023-12-21 14:06:49 浏览: 157
在 .NET 中,可以使用 `System.Security.Cryptography.Crc16` 类来计算字符串的 16 位 CRC 校验码。首先,确保你的项目中已添加对 `System.Security.Cryptography` 命名空间的引用。
以下是一个示例代码:
```csharp
using System;
using System.Security.Cryptography;
using System.Text;
public class CRC16
{
private static ushort[] table = new ushort[256]
{
0x0000, 0xC0C1, 0xC181, 0x0140, 0xC301, 0x03C0, 0x0280, 0xC241,
0xC601, 0x06C0, 0x0780, 0xC741, 0x0500, 0xC5C1, 0xC481, 0x0440,
0xCC01, 0x0CC0, 0x0D80, 0xCD41, 0x0F00, 0xCFC1, 0xCE81, 0x0E40,
0x0A00, 0xCAC1, 0xCB81, 0x0B40, 0xC901, 0x09C0, 0x0880, 0xC841,
// ...
};
public static ushort CalculateCRC(string input)
{
byte[] bytes = Encoding.ASCII.GetBytes(input);
ushort crc = 0xFFFF;
foreach (byte b in bytes)
{
crc = (ushort)((crc >> 8) ^ table[(crc ^ b) & 0xFF]);
}
return crc;
}
}
```
你可以使用以下代码调用 `CRC16.CalculateCRC` 方法来计算字符串的 16 位 CRC 校验码:
```csharp
string input = "Hello, world!";
ushort crc = CRC16.CalculateCRC(input);
Console.WriteLine("CRC16: 0x{0:X4}", crc);
```
这将输出字符串 "Hello, world!" 的 16 位 CRC 校验码。请注意,这里使用了 ASCII 编码,如果你的字符串包含非 ASCII 字符,需要根据实际情况进行编码转换。
阅读全文
相关推荐
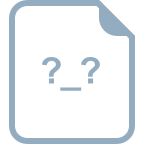
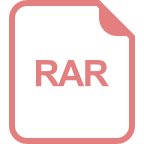
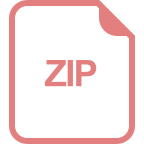
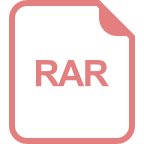
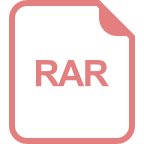
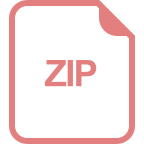
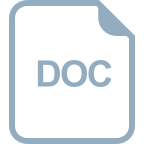
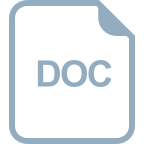

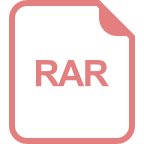
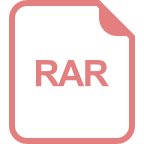
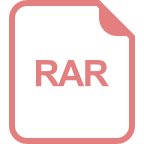
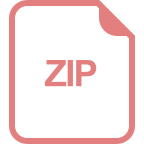
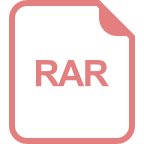
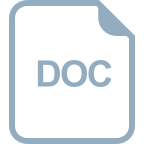
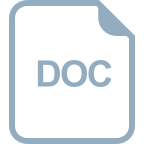