c#打开文件并读取某一行内容
时间: 2024-09-06 17:03:07 浏览: 51
在C#中,打开文件并读取某一行内容通常可以通过`System.IO`命名空间下的类来实现。这里提供一个简单的示例,展示如何使用`StreamReader`类来打开一个文本文件,并读取指定行的内容。
首先,你需要引入`System.IO`命名空间,因为我们需要使用文件操作相关的类:
```csharp
using System;
using System.IO;
```
然后,你可以创建一个方法来打开文件并读取特定行:
```csharp
public static string ReadLineFromFile(string filePath, int lineNumber)
{
string line = null;
try
{
using (StreamReader reader = new StreamReader(filePath))
{
string currentLine;
int currentLineIndex = 0;
while ((currentLine = reader.ReadLine()) != null)
{
currentLineIndex++;
if (currentLineIndex == lineNumber)
{
line = currentLine;
break;
}
}
}
}
catch (Exception ex)
{
Console.WriteLine("Error occurred: " + ex.Message);
}
return line;
}
```
这个`ReadLineFromFile`方法接受文件路径和要读取的行号作为参数。使用`StreamReader`逐行读取文件内容,直到达到指定的行号。
使用方法如下:
```csharp
string path = @"C:\path\to\your\file.txt";
int lineNumber = 5; // 假设我们要读取第5行
string line = ReadLineFromFile(path, lineNumber);
if (line != null)
{
Console.WriteLine("The content of the specified line is: " + line);
}
else
{
Console.WriteLine("The specified line does not exist.");
}
```
确保文件路径正确,并且文件确实存在于该路径下。同时,考虑到文件可能很大,逐行读取是一种效率较高的方式,不会一次性将整个文件加载到内存中。
阅读全文
相关推荐
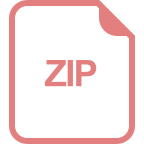
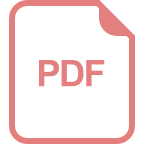
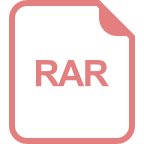



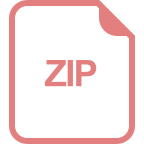
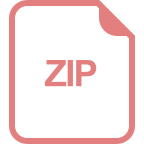
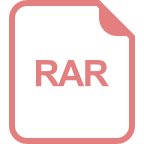
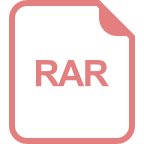
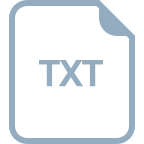
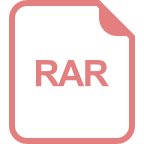






