利用Python以及scapy实现IP存活扫描、端口扫描
时间: 2024-10-13 18:07:09 浏览: 39
在Python中,可以使用`scapy`库来执行IP存活扫描和端口扫描,它是一个非常强大的网络协议分析工具。下面简要概述如何使用`scapy`进行这两项任务:
**IP存活扫描(Pingsweep)**:
```python
from scapy.all import srp, Ether, ARP
def ping(ip):
# 创建ARP请求包
packet = ARP(pdst=ip)
# 发送并获取响应
response = srp(packet, timeout=2, verbose=0)[0]
# 检查是否有回应,如果有的话,IP被认为存活
return response is not None and len(response) > 0
# 对目标列表执行扫描
target_ips = ['192.168.1.1', '10.0.0.2'] # 替换为你想要测试的IP地址
live_ips = [ip for ip in target_ips if ping(ip)]
```
**端口扫描(Portscan)**:
```python
from scapy.all import TCP, sr
def port_scan(ip, ports):
packets = []
for port in ports:
pkt = TCP(sport=RandShort(), dport=port, flags="S")
packets.append(pkt)
answers = sr(packets, timeout=2, verbose=0)
open_ports = []
for sent, received in answers:
if received:
if received[TCP].flags == "SA":
open_ports.append(received[TCP].dport)
return open_ports
# 扫描特定IP范围内的某个端口
ip_to_scan = '192.168.1.1'
ports_of_interest = range(1, 1000) # 可以为你需要扫描的端口号范围
open_ports = port_scan(ip_to_scan, ports_of_interest)
```
**注意事项**:
- `scapy`的操作可能会受到防火墙限制,并且可能被视为潜在的攻击行为,所以在实际使用中请谨慎操作,仅限于合法用途。
-
阅读全文
相关推荐
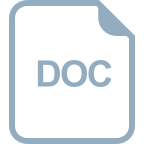
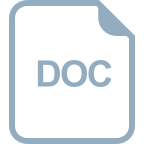
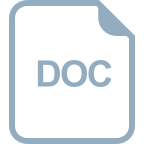



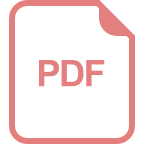
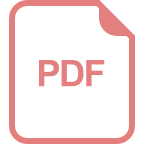
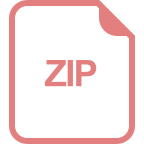
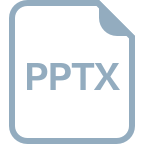
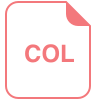
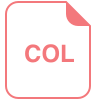
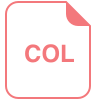
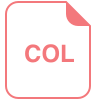
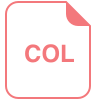
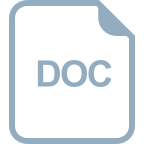
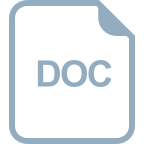
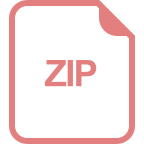
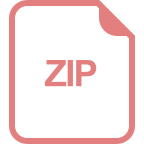