Reorder initializers to match the runtime order.
时间: 2023-12-26 14:20:55 浏览: 131
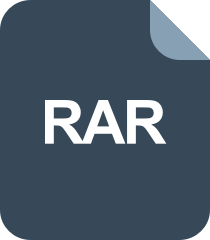
rx_reorder.rar_The Ring_ring buffer
对于调整初始化程序以匹配运行时顺序的问题,您可以按照以下步骤进行操作:
1. 确定运行时顺序:了解程序在运行时期望的初始化顺序。这可能涉及到对象依赖关系,资源分配或其他相关因素。
2. 检查初始化程序:检查现有的初始化程序,了解它们的顺序。
3. 重排序初始化程序:根据运行时顺序的要求重新排列初始化程序。这可能需要修改代码或重新组织初始化代码的结构。
4. 测试和验证:在进行任何更改后,进行测试和验证以确保程序在重新排序后仍然正确运行。
阅读全文
相关推荐
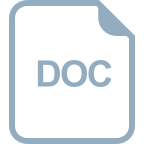
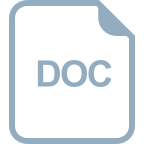
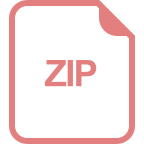
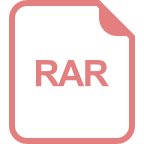
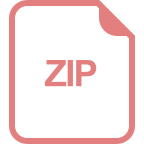
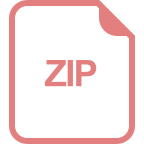
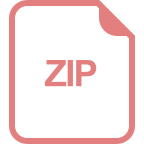
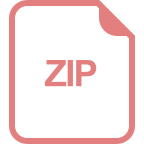









