A store of a final field (inside a constructor) and, if the field is a reference, any store that this final can reference, cannot be reordered with a subsequent store (outside that constructor) of the reference to the object holding that field into a variable accessible to other threads. For example, you cannot reorder
时间: 2024-02-23 18:02:21 浏览: 100
写入 final 字段的操作(在构造函数内部),以及如果该字段是一个引用类型,则任何该 final 引用可以引用的对象的写入操作,都不能与将持有该字段的对象的引用存储到其他线程可访问的变量中(在构造函数外部)的操作进行重排序。
例如,您不能将以下代码进行重排序:
```java
class Example {
final int x;
Object y;
public Example() {
x = 1;
y = new Object();
}
public void publish() {
ExampleHolder.INSTANCE.set(this);
}
}
class ExampleHolder {
static Example INSTANCE;
static void set(Example e) {
INSTANCE = e;
}
}
```
在这个例子中,如果将 `INSTANCE` 的写入操作重排序到 `x` 和 `y` 的写入操作之前,那么在 `publish()` 方法被调用之前,其他线程可能会看到 `INSTANCE` 引用的对象的 `x` 和 `y` 字段为默认值,而不是构造函数中设置的值。这可能会导致程序出现不一致的行为。因此,Java 内存模型禁止这种重排序行为。
相关问题
field a can be final java
在Java中,`final`是一个修饰符,可以用于字段(variable)、方法(method)和类(class)。当你在一个字段上使用`final`,意味着这个字段的值在声明后就不能再改变,即它是常量(constant)。例如:
```java
public class MyClass {
public final int a; // 定义了一个名为a的final整数字段
// constructor
public MyClass(int value) {
this.a = value; // 只能在构造函数中给final字段赋值
}
// 禁止之后修改a的值
public void setA(int b) {
// 这里不允许,因为a已经被标记为final
// this.a = b;
throw new UnsupportedOperationException("Field 'a' is final and cannot be modified.");
}
}
```
这样做的好处有:
1. 提供了代码的确定性和可预测性,尤其是在并发环境中,避免了对共享状态的意外修改。
2. 对于不会变的值,使用final可以提高代码的效率,因为编译器可以做一些优化。
e is a constructor and should be called with the `new` keyword
这个提示意味着你正在尝试调用一个构造函数,但没有使用 `new` 关键字来创建一个新的对象实例。在 JavaScript 中,构造函数必须使用 `new` 关键字来创建一个新的对象实例,否则会出现这个错误。
例如,如果你有一个构造函数 `Person`:
```
function Person(name) {
this.name = name;
}
```
你应该使用 `new` 关键字来创建一个新的 `Person` 对象实例:
```
var person1 = new Person('Alice');
```
如果你没有使用 `new` 关键字,例如这样:
```
var person2 = Person('Bob');
```
那么就会出现 `TypeError: Person is a constructor and should be called with the new keyword` 错误。
阅读全文
相关推荐
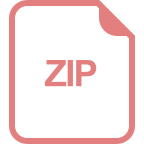
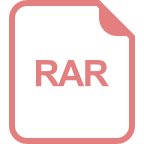
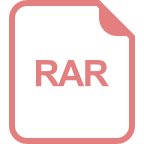













