用队列实现迷宫问题python
时间: 2023-10-13 08:05:40 浏览: 53
NumPy是Python中最基础的数据分析和科学计算库之一。它提供了一个强大的多维数组对象和一系列的数据处理函数,可以有效地进行数值计算和数据分析。通过引用中提供的资源,你可以获得更多关于NumPy的详细信息。
如果你想深入学习NumPy,可以参考试验性的NumPy教程,它提供了一些实践性的练习来帮助你更好地理解NumPy的使用。另外,你也可以查看《从Python到NumPy》以及Matplotlib教程,这些资源都能帮助你更好地掌握NumPy的应用。
如果你在使用NumPy时遇到了问题,可以参考中文官网https://www.numpy.org.cn/来查看API文档,以了解如何正确地导入和使用NumPy库。有时你可能会遇到ModuleNotFoundError: No module named '...'的错误,这可能是因为你没有正确安装NumPy库或者导入时的写法有误。
另外,NumPy还提供了许多有用的统计函数,用于在给定的数组中查找最小值、最大值、标准差和方差等。你可以使用numpy.amin()和numpy.amax()函数来实现这些功能。希望这些信息对你有所帮助!
相关问题
用python队列求解迷宫问题
以下是使用Python队列求解迷宫问题的示例代码:
```python
from queue import Queue
def solve_maze(maze, start, end):
q = Queue()
q.put(start)
visited = set()
while not q.empty():
current = q.get()
if current == end:
return True
visited.add(current)
for direction in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
next_pos = (current[0] + direction[0], current[1] + direction[1])
if next_pos[0] < 0 or next_pos[0] >= len(maze) or next_pos[1] < 0 or next_pos[1] >= len(maze[0]):
continue
if maze[next_pos[0]][next_pos[1]] == 1:
continue
if next_pos in visited:
continue
q.put(next_pos)
return False
maze = [[0, 1, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]]
start = (0, 0)
end = (4, 4)
if solve_maze(maze, start, end):
print("Path found!")
else:
print("Path not found.")
```
该代码使用了队列来实现广度优先搜索算法,从起点开始搜索,每次将当前位置的四个相邻位置加入队列中,直到找到终点或队列为空为止。在搜索过程中,使用一个集合来记录已经访问过的位置,避免重复访问。如果找到了终点,返回True,否则返回False。
迷宫问题python
### 回答1:
迷宫问题可以使用深度优先搜索(DFS)或广度优先搜索(BFS)来解决。这里提供一个基于DFS的Python代码示例:
```python
maze = [[0, 0, 0, 0, 0, 0, 0, 0],
[0, 1, 1, 0, 1, 1, 1, 0],
[0, 1, 0, 0, 0, 1, 0, 0],
[0, 1, 1, 1, 0, 1, 0, 1],
[0, 0, 0, 1, 0, 0, 0, 1],
[0, 1, 0, 0, 0, 1, 1, 1],
[0, 1, 1, 1, 1, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0]]
start = (1, 1)
end = (6, 6)
visited = set()
def dfs(maze, start, end, visited):
if start == end:
return True
visited.add(start)
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
for direction in directions:
next_x = start[0] + direction[0]
next_y = start[1] + direction[1]
if 0 <= next_x < len(maze) and 0 <= next_y < len(maze[0]) and maze[next_x][next_y] == 0 and (next_x, next_y) not in visited:
if dfs(maze, (next_x, next_y), end, visited):
return True
return False
print(dfs(maze, start, end, visited))
```
代码中,`maze`表示迷宫地图,0表示可以通过的通道,1表示障碍物。`start`和`end`分别表示起点和终点位置。`visited`用于记录已经访问过的位置,避免重复访问。
`dfs`函数实现了DFS算法,其中,如果当前位置等于终点,直接返回True;否则将当前位置加入visited集合,遍历四个方向,如果下一个位置在地图范围内、是通道、且没有被访问过,则递归调用dfs函数,如果返回True,则表示找到了一条从起点到终点的路径,直接返回True;否则继续遍历下一个方向。如果所有方向都遍历完了,仍然没有找到路径,则返回False。
最后,在主函数中调用dfs函数,并输出结果。
### 回答2:
迷宫问题是指给定一个迷宫,其中一些位置是墙壁,而其他位置可以通过。我们需要编写一个程序使用Python来找到从起点到终点的最短路径。
首先,我们可以将迷宫表示为一个二维数组或者使用地图数据结构。其中,墙壁用1表示,可以通过的路径用0表示,起点用S表示,终点用E表示。
接下来,我们可以使用深度优先搜索(DFS)或者广度优先搜索(BFS)来解决迷宫问题。下面以广度优先搜索为例来说明。
我们可以定义一个队列来保存将要探索的路径。初始化时,将起点加入队列中。
然后,我们可以使用一个二维数组来保存当前位置的坐标以及到达当前位置所需的步数。初始时,起点的步数为0,其他位置的步数为无穷大。将起点的坐标和步数保存到这个二维数组中。
接下来,我们可以开始探索迷宫。每次从队列中取出一个位置,并根据当前位置的四个相邻位置进行判断。如果相邻位置没有超出迷宫的边界且可以通过(值为0),则将该位置加入队列,并更新到达该位置的步数。
如果终点被加入队列,我们就可以从步数二维数组中找到到达终点的最短路径长度。
最后,我们可以通过回溯从终点回到起点,找到最短路径。从终点开始,依次向前找到步数减一的位置,直到回到起点。期间,我们可以将路径上的坐标保存到一个列表中。
最终,我们可以将得到的最短路径打印出来或者以其他格式呈现。
总结起来,迷宫问题的解决步骤可以概括为:定义起点、终点、迷宫地图。使用广度优先搜索来计算最短路径长度。最后,通过回溯找到最短路径。这个问题可以使用Python编程语言来解决,加深对算法和数据结构的理解。
### 回答3:
迷宫问题是一个经典的算法问题,可以使用Python来解决。
首先,我们可以将迷宫表示为一个二维的数组,其中1表示墙壁,0表示可以通行的路。假设起点在左上角,终点在右下角,我们的目标是找到从起点到终点的路径。
我们可以使用深度优先搜索算法来解决迷宫问题。具体步骤如下:
1. 创建一个辅助函数来进行递归搜索。该函数需要传递当前的位置坐标和访问过的路径。
2. 在递归函数内部,首先判断当前位置是否越界或者为墙壁。如果是,则返回False表示此路不通。
3. 然后判断当前位置是否为终点,如果是,则返回True表示找到了一条通往终点的路径。
4. 如果当前位置既不越界也不为墙壁,那么我们可以将当前位置标记为已访问,并尝试向四个方向(上、下、左、右)进行递归搜索。
5. 如果在四个方向中有一个方向返回True,说明在该方向上找到了通往终点的路径,我们可以立即返回True。
6. 如果四个方向都没有找到通往终点的路径,那么我们需要将当前位置重新标记为未访问,并返回False表示此路不通。
7. 在主函数中,我们调用递归函数,并将起点的位置作为参数传入。如果递归函数返回True,说明找到了通往终点的路径,我们可以打印出路径或者将路径保存起来。
这样,我们就可以使用Python解决迷宫问题了。实际上,这只是深度优先搜索的一种简单应用,还有其他更高效的算法可以解决迷宫问题,比如广度优先搜索或者使用A*算法。对于更复杂的迷宫,我们可能需要使用更复杂的算法来解决。
相关推荐
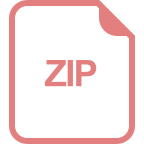
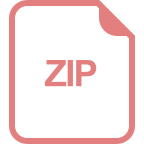
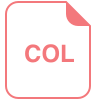
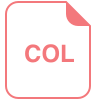
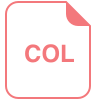
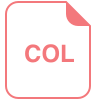
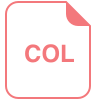







