汉诺塔非递归c语言代码
时间: 2023-10-12 18:05:14 浏览: 98
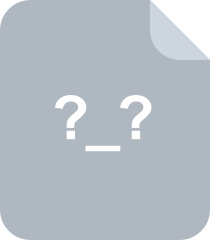
汉诺塔非递归C语言实现.c

以下是汉诺塔的非递归C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
// 定义堆栈结构
struct Stack {
int capacity;
int top;
int *array;
};
// 创建堆栈
struct Stack *createStack(int capacity) {
struct Stack *stack = (struct Stack *)malloc(sizeof(struct Stack));
stack->capacity = capacity;
stack->top = -1;
stack->array = (int *)malloc(stack->capacity * sizeof(int));
return stack;
}
// 判断堆栈是否为空
int isEmpty(struct Stack *stack) {
return stack->top == -1;
}
// 判断堆栈是否已满
int isFull(struct Stack *stack) {
return stack->top == stack->capacity - 1;
}
// 入栈
void push(struct Stack *stack, int item) {
if (isFull(stack)) {
return;
}
stack->array[++stack->top] = item;
}
// 出栈
int pop(struct Stack *stack) {
if (isEmpty(stack)) {
return -1;
}
return stack->array[stack->top--];
}
// 非递归汉诺塔函数
void hanoi(int n) {
struct Stack *source = createStack(n);
struct Stack *auxiliary = createStack(n);
struct Stack *destination = createStack(n);
int i;
for (i = n; i > 0; i--) {
push(source, i);
}
int totalMoves = (1 << n) - 1;
int from, to;
if (n % 2 == 0) {
from = 2;
to = 3;
} else {
from = 1;
to = 2;
}
for (i = 1; i <= totalMoves; i++) {
if (i % 3 == 1) {
if (isEmpty(destination)) {
push(destination, pop(source));
} else if (isEmpty(source)) {
push(source, pop(destination));
} else if (source->array[source->top] > destination->array[destination->top]) {
push(destination, pop(source));
} else {
push(source, pop(destination));
}
} else if (i % 3 == 2) {
if (isEmpty(auxiliary)) {
push(auxiliary, pop(source));
} else if (isEmpty(source)) {
push(source, pop(auxiliary));
} else if (source->array[source->top] > auxiliary->array[auxiliary->top]) {
push(auxiliary, pop(source));
} else {
push(source, pop(auxiliary));
}
} else if (i % 3 == 0) {
if (isEmpty(destination)) {
push(destination, pop(auxiliary));
} else if (isEmpty(auxiliary)) {
push(auxiliary, pop(destination));
} else if (auxiliary->array[auxiliary->top] > destination->array[destination->top]) {
push(destination, pop(auxiliary));
} else {
push(auxiliary, pop(destination));
}
}
printf("Move disk %d from rod %d to rod %d\n", i, from, to);
if (from == 1 && to == 2) {
from = 1;
to = 3;
} else if (from == 1 && to == 3) {
from = 2;
to = 3;
} else {
from = 1;
to = 2;
}
}
}
// 主函数
int main() {
int n = 3;
hanoi(n);
return 0;
}
```
阅读全文
相关推荐


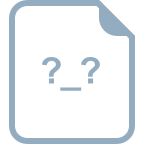
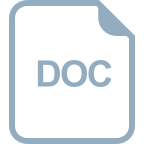

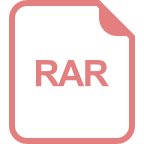
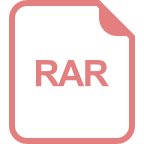
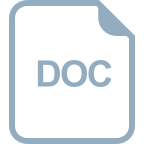
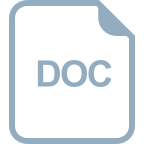
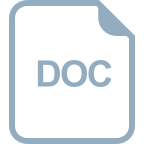
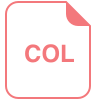

