为什么在qt中用不了VideoCapture类的getDevices函数
时间: 2023-05-21 12:02:19 浏览: 212
可能是因为 VideoCapture 类在 Qt 中没有被正确地初始化或者没有被正确地链接到 OpenCV 库。您可以尝试在 Qt 项目中添加 OpenCV 库并重新编译项目,或者使用其他可用的方法来获取摄像头设备列表。
相关问题
帮我写一个关于VideoCapture类cvtColor函数goodFeaturesToTrack函数得实验总结
好的,下面是关于 `VideoCapture` 类、`cvtColor` 函数和 `goodFeaturesToTrack` 函数的实验总结。
## 实验目的
1. 掌握 `VideoCapture` 类的基本功能和使用方法;
2. 掌握 `cvtColor` 函数的基本功能和使用方法;
3. 掌握 `goodFeaturesToTrack` 函数的基本功能和使用方法。
## 实验环境
- 操作系统:Windows 10;
- 开发工具:Visual Studio 2019;
- 编程语言:C++;
- 库:OpenCV 4.5.3。
## 实验内容
### 1. 读取视频文件并显示
首先,我们使用 `VideoCapture` 类读取一段视频文件,并将读取到的帧显示出来。代码如下:
```c++
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
VideoCapture cap("test.mp4"); // 打开视频文件
if(!cap.isOpened())
{
printf("Failed to open video file!\n");
return -1;
}
namedWindow("Video", WINDOW_NORMAL);
while(true)
{
Mat frame;
if(!cap.read(frame)) // 读取一帧数据
break;
imshow("Video", frame); // 显示帧
if(waitKey(30) == 27) // 按 ESC 键退出
break;
}
cap.release(); // 释放资源
destroyAllWindows();
return 0;
}
```
在程序中,我们首先打开视频文件,如果打开失败则退出程序。然后创建一个窗口用于显示视频帧,并且不断读取视频文件中的帧并显示出来,直到视频文件读取完毕或者用户按下 ESC 键退出程序。
### 2. 颜色空间转换
接下来,我们使用 `cvtColor` 函数将一张彩色图片转换为灰度图片。代码如下:
```c++
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat image = imread("test.jpg");
if(image.empty())
{
printf("Failed to open image file!\n");
return -1;
}
Mat gray;
cvtColor(image, gray, COLOR_BGR2GRAY); // BGR -> 灰度
namedWindow("Image", WINDOW_NORMAL);
namedWindow("Gray", WINDOW_NORMAL);
imshow("Image", image);
imshow("Gray", gray);
waitKey(0);
destroyAllWindows();
return 0;
}
```
在程序中,我们首先读取一张彩色图片,如果读取失败则退出程序。然后使用 `cvtColor` 函数将彩色图片转换为灰度图片,并将结果显示出来。
### 3. 关键点检测
最后,我们使用 `goodFeaturesToTrack` 函数检测一张图片中的关键点。代码如下:
```c++
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat image = imread("test.jpg");
if(image.empty())
{
printf("Failed to open image file!\n");
return -1;
}
Mat gray;
cvtColor(image, gray, COLOR_BGR2GRAY); // BGR -> 灰度
vector<Point2f> corners;
goodFeaturesToTrack(gray, corners, 200, 0.01, 10); // 检测关键点
for(size_t i = 0; i < corners.size(); i++)
{
circle(image, corners[i], 5, Scalar(0, 0, 255), 2); // 画圆
}
namedWindow("Image", WINDOW_NORMAL);
imshow("Image", image);
waitKey(0);
destroyAllWindows();
return 0;
}
```
在程序中,我们首先读取一张图片,如果读取失败则退出程序。然后将图片转换为灰度图片,并使用 `goodFeaturesToTrack` 函数检测图片中的关键点。最后,我们将关键点用红色圆圈标识出来,并将结果显示出来。
## 实验总结
本次实验中,我们学习了 `VideoCapture` 类、`cvtColor` 函数和 `goodFeaturesToTrack` 函数的基本功能和使用方法,并且通过实验加深了对它们的理解。在实际应用中,我们可以根据需要使用它们来处理视频和图像。
VideoCapture 类在 Qt 中怎么初始化
VideoCapture 类在 Qt 中的初始化方式如下:
1. 首先,需要在代码中包含头文件 #include <opencv2/opencv.hpp>。
2. 然后,定义一个 VideoCapture 对象,例如:
cv::VideoCapture cap;
3. 接着,调用 cap.open() 方法打开摄像头或者视频文件,例如:
cap.open(0); // 打开默认摄像头
cap.open("test.mp4"); // 打开视频文件
4. 最后,可以使用 cap.read() 方法读取视频帧,例如:
cv::Mat frame;
cap.read(frame); // 读取一帧视频
注意:在使用 VideoCapture 类时,需要保证 OpenCV 库已经正确安装并链接到项目中。
阅读全文
相关推荐
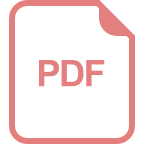
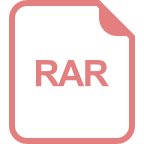










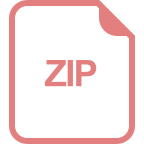